There are four different methods to add a title to seaborn plots. Let us explore each of these methods in detail with examples.
Method 1: Using set() method
The set()
method is used to add different elements to the plot, and we can use it to add the title by using the title parameter in the case of a single seaborn plot.
# import pandas library
import pandas as pd
from pyparsing import line
import seaborn as sns
import matplotlib.pyplot as plt
# create pandas DataFrame
df = pd.DataFrame({'team': ['India', 'South Africa', 'New Zealand', 'England'],
'points': [10, 8, 3, 5],
'runrate': [0.5, 1.4, 2, -0.6],
'wins': [5, 4, 2, 2]})
# plot the data frame
line_plt = sns.lineplot(data = df)
line_plt.set(title = "ICC World Cup Standings")
plt.show()
Output
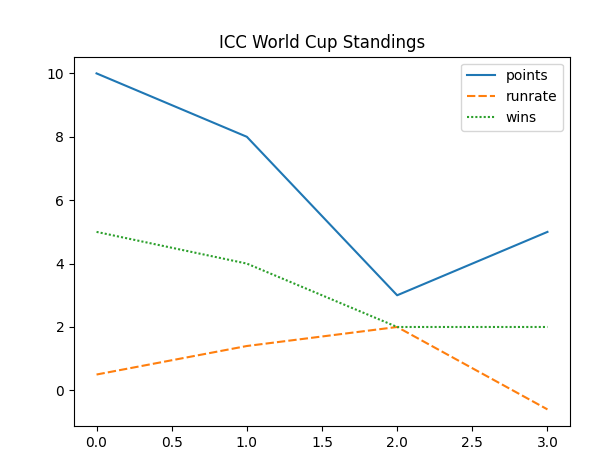
Method 2: Using set_title() method
The seaborn plot returns an instance of Matplotlib axes, and for such objects, we can use the set_title()
method, which accepts one parameter title as an argument.
# import pandas library
import pandas as pd
from pyparsing import line
import seaborn as sns
import matplotlib.pyplot as plt
# create pandas DataFrame
df = pd.DataFrame({'team': ['India', 'South Africa', 'New Zealand', 'England'],
'points': [10, 8, 3, 5],
'runrate': [0.5, 1.4, 2, -0.6],
'wins': [5, 4, 2, 2]})
# plot the data frame
line_plt = sns.lineplot(data = df)
line_plt.set_title("ICC World Cup Standings")
plt.show()
Output
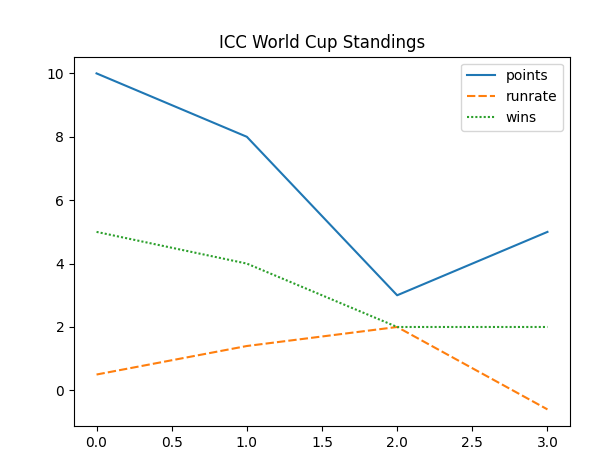
Method 3: Using title() method
We can also leverage matplotlib.pyplot.title()
method, which offers a lot of customization such as location, color, font size, etc.
# import pandas library
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
# create pandas DataFrame
df = pd.DataFrame({'team': ['India', 'South Africa', 'New Zealand', 'England'],
'points': [10, 8, 3, 5],
'runrate': [0.5, 1.4, 2, -0.6],
'wins': [5, 4, 2, 2]})
# plot the data frame
line_plt = sns.lineplot(data = df)
# set title using matplotlib title
plt.title("ICC World Cup Standings")
plt.show()
Output
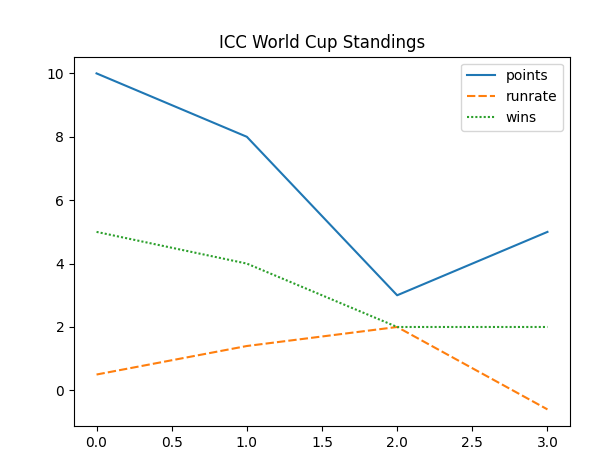
Method 4: Using suptitle() method
We can also use suptitle()
method to add an overall title to the seaborn plot. This is mainly useful if you have many subplots, but you still need to use one single title to represent the charts.
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
# create pandas DataFrame
df = pd.DataFrame({'wins': [12, 11, 10, 3, 11, 20, 2, 30, 12,7],
'lost': [6, 4, 5, 3, 10, 7, 2, 12, 0, 6],
'team': ['A', 'A', 'A', 'A', 'A', 'B', 'B', 'B', 'B', 'B']})
# plot the data frame
rel = sns.relplot(data=df, x='wins', y='lost', col='team')
# add overall title
rel.fig.suptitle('ICC World Cup Standings')
plt.show()
Output
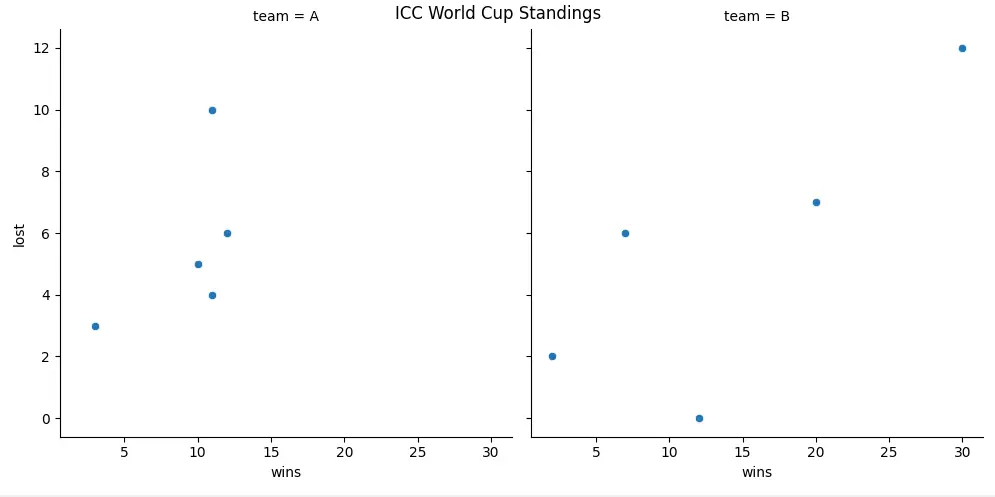
from Planet Python
via read more
No comments:
Post a Comment