Welcome to another module of TypeError in the python programming language. In today’s article, we will be discussing an embarrassing Typeerror that usually gets landed up while we are a beginner to python. The error is named as TypeError: ‘method’ object is not subscriptable Solution.
In this guide, we discuss what this error means and why you may encounter it. Then, we walk through an example of this error to help you develop a solution.
What are subscriptable objects in python?
Subscriptable objects are objects with a __getitem__ method. These are the data types such as lists, dictionaries, and tuples. The __getitem__ method allows the Python interpreter to retrieve an individual item from a collection.
Not all objects are subscriptable. Methods, for instance, are not. This is because they do not implement the __getitem__ method. This means you cannot use square bracket syntax to access the items in a method or call a method.
Why do you get TypeError: ‘method’ object is not subscriptable Solution in python?
Arguments in Python methods should be specified within parentheses. This is because functions and methods both use parentheses to tell if they are being called. If you use square brackets to call a method, you will encounter a “TypeError: ‘method’ object is not subscriptable” error.
Let us consider the following code snippet:
names = ["Python", "Pool", "Latracal", "Google"]
print(names[0])
OUTPUT:- Python
This code returns “Python,” the name at the index position 0. We cannot use square brackets to call a function or a method because functions and methods are not subscriptable objects.
Code for the TypeError
class Names:
def __init__(self, name, birth):
self.name = name
self.birth = birth
def get_country(self, to_compare):
if to_compare == self.birth:
print("{} is from {}.".format(self.name, self.birth))
else:
print("{} is not from {}. She is from {}.".format(
self.name, to_compare, self.birth))
kimmi = Names("kimmi", "India")
kimmi.get_country["Germany"]
OUTPUT:-
![[Solved] TypeError: ‘method’ Object is not ubscriptable](https://www.pythonpool.com/wp-content/uploads/2021/05/Untitled-1.png)
Explanation of the code
- We build a program that stores names in objects.
- Here the “Names” class that we use to define a name will have a method that lets us check whether that person is from a particular country of birth.
- Our class contains two methods, __init__ and get_Country.
- The first method defines the structure of the Name object.
- The second lets us check whether the country of birth of the person is equal to a particular value.
- Next, we create an object from our Name class.
- The variable “Kimmi” is an object. The name associated with the name is Kimmi, and its country of birth is “India”.
- Next, let’s call our
get_country()
method. - This code executes the
get_country()
method from the Names class. Theget_country()
method checks whether the value of “birth” in our “Kimmi” object is equal to “Germany”. - And thus, an error occurs in our code.
The solution to the TypeError: method Object is not Subscriptable
Let us first analyze the line of code that the Python debugger has identified as TypeError:
Kimmi.get_country["Germany"]
In this line of code, we used square brackets to call the get_country()
method. This is not an acceptable syntax in python because square brackets are used to access items from a list. Because functions and objects are not subscriptable, we cannot use square brackets to call them.
So to solve this error, we must replace the square brackets with round brackets:
Kimmi.get_country("Germany")
Let us complete the code:
class Names:
def __init__(self, name, birth):
self.name = name
self.birth = birth
def get_country(self, to_compare):
if to_compare == self.birth:
print("{} is from {}.".format(self.name, self.birth))
else:
print("{} is not from {}. She is from {}.".format(
self.name, to_compare, self.birth))
kimmi = Names("kimmi", "India")
kimmi.get_country("Germany")
OUTPUT:-

Our code works if the value we specify is equal to the country of birth of the person.
Also, Read
FAQs
1. When do we get TypeError: ‘builtin_function_or_method’ object is not subscriptable?
Ans:- Let us look as the following code snippet first to understand this.
import numpy as np
a = np.array[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
OUTPUT:-
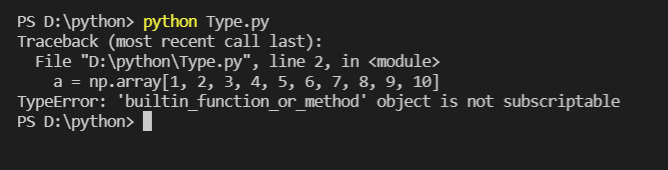
This problem is usually caused by missing the round parentheses in the np.array line.
It should have been written as:
a = np.array([1,2,3,4,5,6,7,8,9,10])
It is quite similar to TypeError: ‘method’ object is not subscriptable the only difference is that here we are using a library numpy so we get TypeError: ‘builtin_function_or_method’ object is not subscriptable.
Conclusion
The “TypeError: ‘method’ object is not subscriptable” error is raised when you use square brackets to call a method inside a class. To solve this error, make sure that you only call methods of a class using round brackets after the name of the method you want to call.
Now you’re ready to solve this common Python error like a professional coder! Till then keep pythoning Geeks!
The post [Solved] TypeError: method Object is not Subscriptable appeared first on Python Pool.
from Planet Python
via read more
No comments:
Post a Comment