Friday, May 31, 2019
Peter Bengtsson: Generate a random IP address in Python
from Planet Python
via read more
Davy Wybiral: Always Secure Your localhost Servers
You should see "Found: HTTP (8080)" listed and that's because the Javascript on that page made an HTTP GET request to your local server to determine that it was running. Chances are it detected other services as well (for instance if you run Tor or Keybase locally).
There are two implications from this that follow:
- Website owners could potentially use this to collect information about what popular services are running on your local network.
- Malicious actors could use this to exploit vulnerabilities in those services.
For instance, at one point Redis was vulnerable to these types of attacks because its protocol is text-over-TCP (just like HTTP) so any web page you visit could craft an HTTP POST request that mimics normal Redis commands. There's an excellent write-up on this vulnerability here (which has since been fixed).
Similarly, if you run the popular gaming platform Steam on Linux, at the time of writing this the main application opens a TCP listener on port 57343. I have no idea what that port is for but I do know that visiting this web page while Steam is open will cause the entire UI to freeze, as well as most games, until the tab is closed: [WARNING: don't blame me if it crashes your game] https://wybiral.github.io/steam-block/
This works because the favicon on that page is actually an HTTP GET request to the TCP server which never closes, thus blocking their listener. It may even be possible to attack the server in other ways with specifically crafted requests (I was able to crash the application using a large enough payload).
These types of vulnerabilities are widespread mostly because application developers assume that the server will only be locally-accessible and not available to every website the user visits. Hopefully this is enough proof to justify why better security measures need to be taken with local servers even if you don't intend to forward the ports you're using to the outside world.
So, as developers what can we do to prevent this kind of attack?
For HTTP and WebSocket servers you can add authentication, CSRF protection, and restrict access based on the page origin (which browsers should include in the request headers).
For TCP servers it could be harder depending on your application. You can detect HTTP-like request headers and block the connection (this seems to be what Redis is doing now). Or require some sort of handshake/authentication that the browser won't perform and reject connections based on that.
As far as preventing fingerprinting and service discovery this way... I'm not entirely sure what the best way to prevent this would be other than ungracefully failing the requests (which a lot of HTTP frameworks probably don't make easy). If anyone has any ideas here feel free to drop me a tweet @davywtf.
from Planet Python
via read more
Davy Wybiral: Always Secure Your localhost Servers
You should see "Found: HTTP (8080)" listed and that's because the Javascript on that page made an HTTP GET request to your local server to determine that it was running. Chances are it detected other services as well (for instance if you run Tor or Keybase locally).
There are two implications from this that follow:
- Website owners could potentially use this to collect information about what popular services are running on your local network.
- Malicious actors could use this to exploit vulnerabilities in those services.
For instance, at one point Redis was vulnerable to these types of attacks because its protocol is text-over-TCP (just like HTTP) so any web page you visit could craft an HTTP POST request that mimics normal Redis commands. There's an excellent write-up on this vulnerability here (which has since been fixed).
Similarly, if you run the popular gaming platform Steam on Linux, at the time of writing this the main application opens a TCP listener on port 57343. I have no idea what that port is for but I do know that visiting this web page while Steam is open will cause the entire UI to freeze, as well as most games, until the tab is closed: [WARNING: don't blame me if it crashes your game] https://wybiral.github.io/steam-block/
This works because the favicon on that page is actually an HTTP GET request to the TCP server which never closes, thus blocking their listener. It may even be possible to attack the server in other ways with specifically crafted requests (I was able to crash the application using a large enough payload).
These types of vulnerabilities are widespread mostly because application developers assume that the server will only be locally-accessible and not available to every website the user visits. Hopefully this is enough proof to justify why better security measures need to be taken with local servers even if you don't intend to forward the ports you're using to the outside world.
So, as developers what can we do to prevent this kind of attack?
For HTTP and WebSocket servers you can add authentication, CSRF protection, and restrict access based on the page origin (which browsers should include in the request headers).
For TCP servers it could be harder depending on your application. You can detect HTTP-like request headers and block the connection (this seems to be what Redis is doing now). Or require some sort of handshake/authentication that the browser won't perform and reject connections based on that.
As far as preventing fingerprinting and service discovery this way... I'm not entirely sure what the best way to prevent this would be other than ungracefully failing the requests (which a lot of HTTP frameworks probably don't make easy). If anyone has any ideas here feel free to drop me a tweet @davywtf.
from Planet Python
via read more
Stack Abuse: The Python String strip() Function
In this article, we'll examine how to strip characters from both ends of a string in Python.
The built-in String
type is an essential Python structure, and comes with a built-in set of methods to simplify working with text data. There are many situations in which a programmer may want to remove unwanted characters, i.e. strip certain characters, from either the beginning or ending of a string.
The most common requirement is to strip whitespace (spaces, tabs, newline characters, etc.) from both ends of a string. This usually occurs after importing raw text data from a file, database, web service, or after accepting user input, which may contain typos in the form of extra spaces. This can be handled by the default usage of the String.strip()
method, as seen here:
>>> orig_text = ' The cow jumped over the moon! \n'
>>> print(orig_text.strip())
The cow jumped over the moon!
>>>
Note that this method does not change the original value of the string, i.e. it does not change the string in-place. It simply returns a new string with the whitespace on either end stripped out. We can verify this by printing out the original string:
>>> print(orig_text)
The cow jumped over the moon!
>>>
The strip
method also enables us to specify which types of characters we want to strip. This can be useful if we want to remove other characters besides whitespace. To do this we simply specify the characters to strip by passing an argument containing these characters to the String.strip()
method:
>>> orig_text = '-----The cow jumped over the moon!$$$$$'
>>> print(orig_text.strip('-$'))
The cow jumped over the moon!
>>>
This is useful for removing characters at the start or end of a string that were used for formatting purposes, for example. So if you have a Markdown-formatted string, you can easily remove the header syntax like this:
>>> md_text = '### My Header Here' # Denotes an H3 header in Markdown
>>> print(md_text.strip('# '))
My Header Here
>>>
Finally, Python provides a way to strip characters from only one side of the string via the String.rstrip()
and String.lstrip()
methods. These methods work exactly the same way as the String.strip()
method, but String.rstrip()
only removes characters from the right side of the string and String.lstrip()
only removes characters from the left side of the string:
>>> orig_text = '*****The cow jumped over the moon!*****'
>>> print(orig_text.rstrip('*'))
*****The cow jumped over the moon!
>>> print(orig_text.lstrip('*'))
The cow jumped over the moon!*****
Once again we can print the original string to see that it was unaffected by these operations:
>>> print(orig_text)
*****The cow jumped over the moon!*****
`
About the Author
This article was written by Jacob Stopak, a software consultant and developer with passion for helping others improve their lives through code. Jacob is the creator of Initial Commit - a site dedicated to helping curious developers learn how their favorite programs are coded. Its featured project helps people learn Git at the code level.
from Planet Python
via read more
Talk Python to Me: #214 Dive into CPython 3.8 and beyond
from Planet Python
via read more
Stack Abuse: The Python zip() Function
In this article, we'll examine how to use the built-in Python zip()
function.
The zip()
function is a Python built-in function that allows us to combine corresponding elements from multiple sequences into a single list of tuples. The sequences are the arguments accepted by the zip()
function. Any number of sequences can be supplied, but the most common use-case is to combine corresponding elements in two sequences.
For example, let's say we have the two lists below:
>>> vehicles = ['unicycle', 'motorcycle', 'plane', 'car', 'truck']
>>> wheels = [1, 2, 3, 4, 18]
We can use the zip()
function to associate elements from these two lists based on their order:
>>> list(zip(vehicles, wheels))
[('unicycle', 1), ('motorcycle', 2), ('plane', 3), ('car', 4), ('truck', 18)]
Notice how the output is a sequence of tuples, where each tuple combines elements of the input sequences with corresponding indexes.
One important thing to note is that if the input sequences are of differing lengths, zip()
will only match elements until the end of the shortest list is reached. For example:
>>> vehicles = ['unicycle', 'motorcycle', 'plane', 'car', 'truck']
>>> wheels = [1, 2, 3]
>>> list(zip(vehicles, wheels))
[('unicycle', 1), ('motorcycle', 2), ('plane', 3)]
Since the wheels
list is shorter in this example (3 items as opposed to the 5 that vehicles
has), the sequence stopped at "plane".
As previously mentioned, the zip()
function can be used with more than two sequences:
>>> vehicles = ['unicycle', 'motorcycle', 'plane', 'car', 'truck']
>>> wheels = [1, 2, 3, 4, 18]
>>> energy_sources = ['pedal', 'gasoline', 'jet fuel', 'gasoline', 'diesel']
>>> list(zip(vehicles, wheels, energy_sources))
[('unicycle', 1, 'pedal'), ('motorcycle', 2, 'gasoline'), ('plane', 3, 'jet fuel'), ('car', 4, 'gasoline'), ('truck', 18, 'diesel')]
One reason to connect multiple sequences like this is to create a cleaner way to iterate over the items in multiple sequences. Without the zip()
function, we would have to do something like this:
>>> for i in range(len(vehicles)):
... print('A ' + vehicles[i] + ' has ' + str(wheels[i]) + ' wheels and runs on ' + energy_sources[i])
...
A unicycle has 1 wheels and runs on pedal
A motorcycle has 2 wheels and runs on gasoline
A plane has 3 wheels and runs on jet fuel
A car has 4 wheels and runs on gasoline
A truck has 18 wheels and runs on diesel
But with the zip()
function we can use the following cleaner syntax via tuple unpacking:
>>> for v, w, es in zip(vehicles, wheels, energy_sources):
... print('A ' + v + ' has ' + str(w) + ' wheels and runs on ' + es)
...
A unicycle has 1 wheels and runs on pedal
A motorcycle has 2 wheels and runs on gasoline
A plane has 3 wheels and runs on jet fuel
A car has 4 wheels and runs on gasoline
A truck has 18 wheels and runs on diesel
One final thing to understand about the zip()
function is that it actually returns an iterator, not a list of tuples. Note that in our first two examples above, we wrapped the zip()
function inside the list()
type to convert the result to a list. If we tried to display the return value of the zip()
function directly we would see something like this:
>>> zip(vehicles, wheels)
<zip object at 0x1032caf48>
This 'zip object' is an iterable instance of the Zip
class, which means it will return its contents one by one in a for-loop, instead of all at once, the way a list does. This is more efficient for large sequences that would be very memory intensive if accessed all at once.
About the Author
This article was written by Jacob Stopak, a software consultant and developer with passion for helping others improve their lives through code. Jacob is the creator of Initial Commit - a site dedicated to helping curious developers learn how their favorite programs are coded. Its featured project helps people learn Git at the code level.
from Planet Python
via read more
Quansight Labs Blog: metadsl: A Framework for Domain Specific Languages in Python
metadsl
: A Framework for Domain Specific Languages in Python¶
Hello, my name is Saul Shanabrook and for the past year or so I have been at Quansight exploring the array computing ecosystem. This started with working on the xnd project, a set of low level primitives to help build cross platform NumPy-like APIs, and then started exploring Lenore Mullin's work on a mathematics of arrays. After spending quite a bit of time working on an integrated solution built on these concepts, I decided to step back to try to generalize and simplify the core concepts. The trickiest part was not actually compiling mathematical descriptions of array operations in Python, but figuring out how to make it useful to existing users. To do this, we need to meet users where they are at, which is with the APIs they are already familiar with, like numpy
. The goal of metadsl
is to make it easier to tackle parts of this problem seperately so that we can collaborate on tackling it together.
Libraries for Scientific Computing¶
Much of the recent rise of Python's popularity is due to its usage for scientific computing and machine learning. This work is built on different frameworks, like Pandas, NumPy, Tensorflow, and scikit-learn. Each of these are meant to be used from Python, but have their own concepts and abstractions to learn on top of the core language, so we can look at them as Domain Specific Languages (DSLs). As the ecosystem has matured, we are now demanding more flexibility for how these languages are executed. Dask gives us a way to write Pandas or NumPy and execute it across many cores or computers, Ibis allows us to write Pandas but on a SQL database, with CuPy we can execute NumPy on our GPU, and with Numba we can optimize our NumPy expession on a CPU or GPU. These projects prove that it is possible to write optimizing compilers that target varying hardware paradigms for existing Python numeric APIs. However, this isn't straightforward and these projects success is a testament to the perserverence and ingenuity of the authors. We need to make it easy to add reusable optimizations to libraries like these, so that we can support the latest hardware and compiler optimizations from Python. metadsl
is meant to be a place to come together to build a framework for DSLs in Python. It provides a way to seperate the user experience from the the specific of execution, to enable consistency and flexibility for users. In this post, I will go through an example of creating a very basic DSL. It will not use the metadsl
library, but will created in the same style as metadsl
to illustrate its basic principles.
Read more… (4 min remaining to read)
from Planet Python
via read more
Wingware Blog: Using External Code Quality Checkers with Wing Pro 7
Wing Pro 7 introduced a new code warnings system with expanded built-in code inspection capabilities and improved integration with external code quality checkers. In this issue of Wing Tips, we describe how to configure Wing Pro 7 to take advantage of external code quality checkers like Pylint, pep8, and mypy.
Pylint is a powerful general-purpose code quality checker that flags a wide variety of real code errors and stylistic issues, pep8 checks compliance with the PEP 8 Style Guide for Python Code, and mypy is a type checker for Python 3 code that makes use of optional static type declarations.
Installing the External Checkers
If you need to install Pylint, pep8, or mypy, you can do this on the command line outside of Wing like this:
pip install pylint
Or if you are using Anaconda, you should use conda instead:
conda install pylint
The package name for the other two checkers are pep8 and mypy.
A quick way to see whether the checkers are properly installed is to attempt to import them in Wing's Python Shell tool:
>>> import pylint >>> import pep8 >>> import mypy
If you need to change which Python installation Wing uses, set the Python Executable under the Environment tab in Project Properties, from the Project menu, to the value produced by import sys; print(sys.executable) in the Python interpreter that you want to use. Then restart Wing's Python Shell from its Options menu.
Configuring External Checkers
Once the checkers you plan to use are installed, you can enable them in Wing Pro's Code Warnings tool, from the Tools menu. Click on the Configuration tab, check on Enable external checkers, and then press the Configure button. This displays a dialog like this:
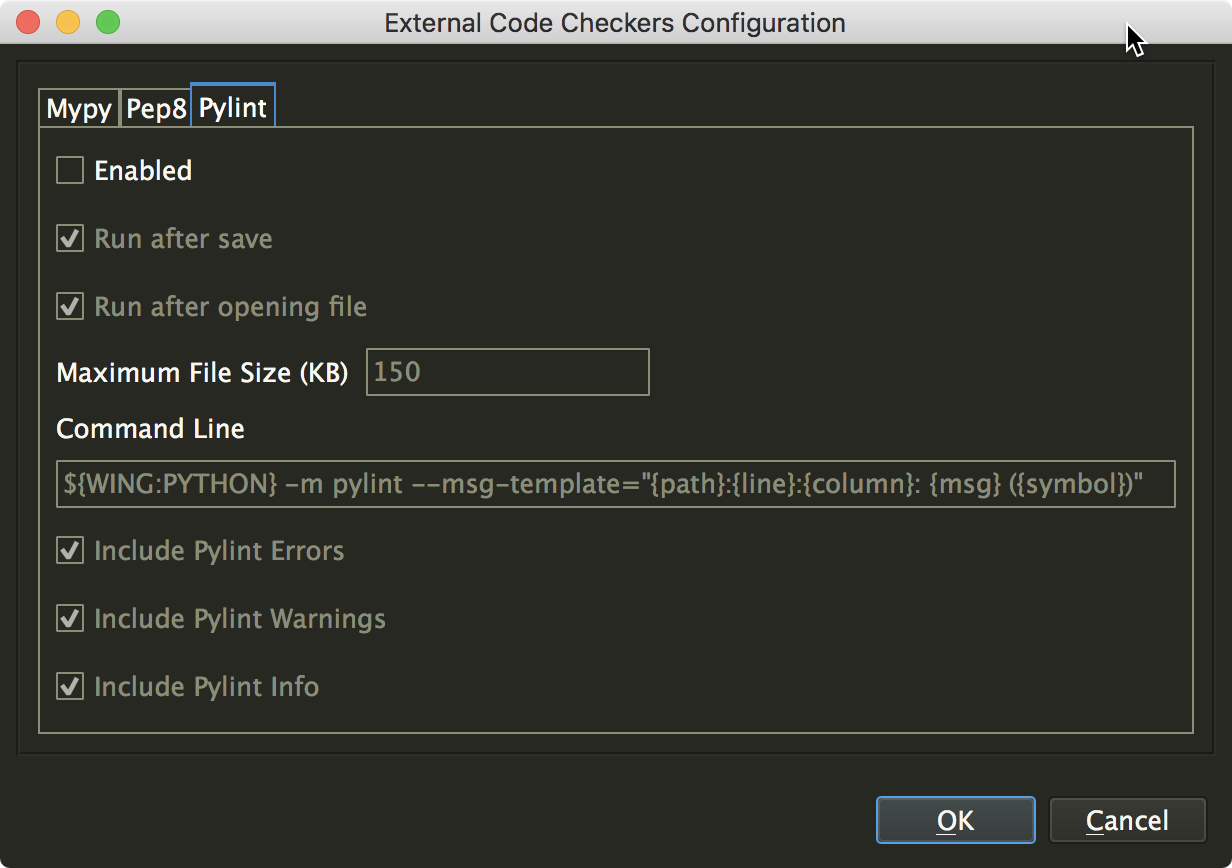
Here you can check on Enable for each of the external checkers that you want to use with your project, and select some options for when and how they are run. In most cases the default configuration will work. Wing will run the checker when a file is opened and re-run it each time the file is saved. For Pylint, you can select whether Wing will display only errors, or also warnings and informational messages.
Notice that Wing avoids running the checkers on source files above the configured Maximum File Size, in order to avoid overly lengthy CPU-intensive computation.
Using the Checkers
Once this is done, Wing will interleave errors and warnings found by the enabled checkers into its own warnings in the Code Warnings tool:
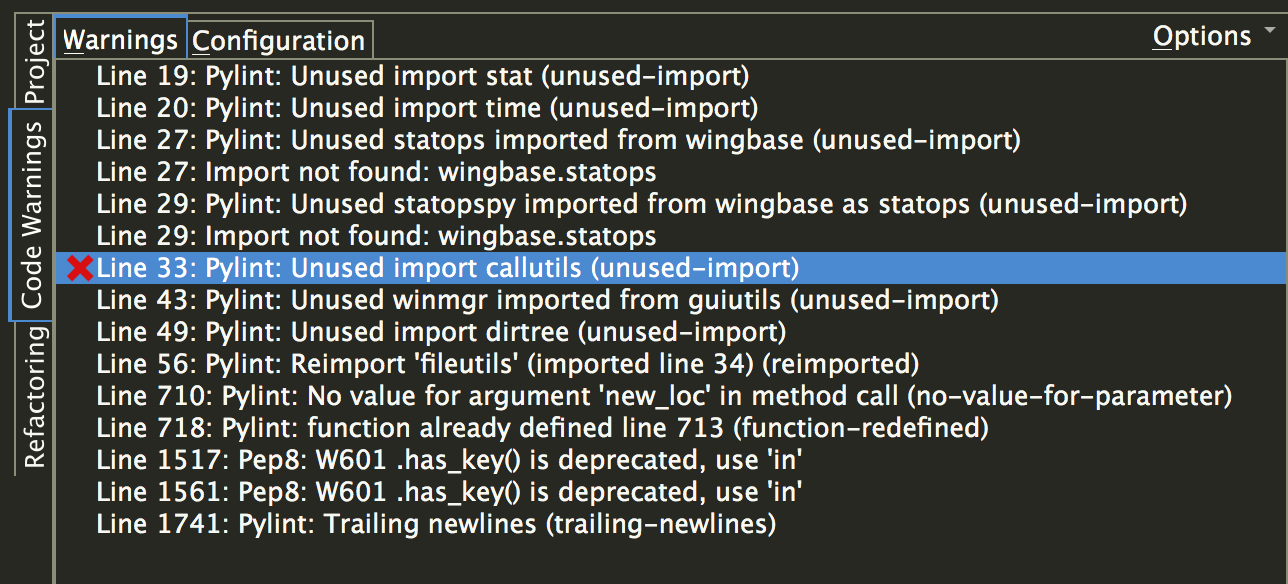
Warnings are also shown on the editor, using indicators that can be configured with the Editor > Code Warnings preferences group. Hovering the mouse over an indicator in the editor displays the warning in a tooltip:

Filtering Out Warnings
Checkers like Pylint can produce lots of stylistic warnings that may not be relevant to your development team's coding standards. These can be a distraction, making it harder to see real problems in code.
Wing lets you quickly hide incorrect or uninteresting warnings by clicking on the red icon that appears when you mouse over items in the Code Warnings tool.
For external checkers like Pylint and pep8, this hides all warnings of that type, by adding a rule to Removed from any file section under the Configuration tab in the Code Warnings tool:
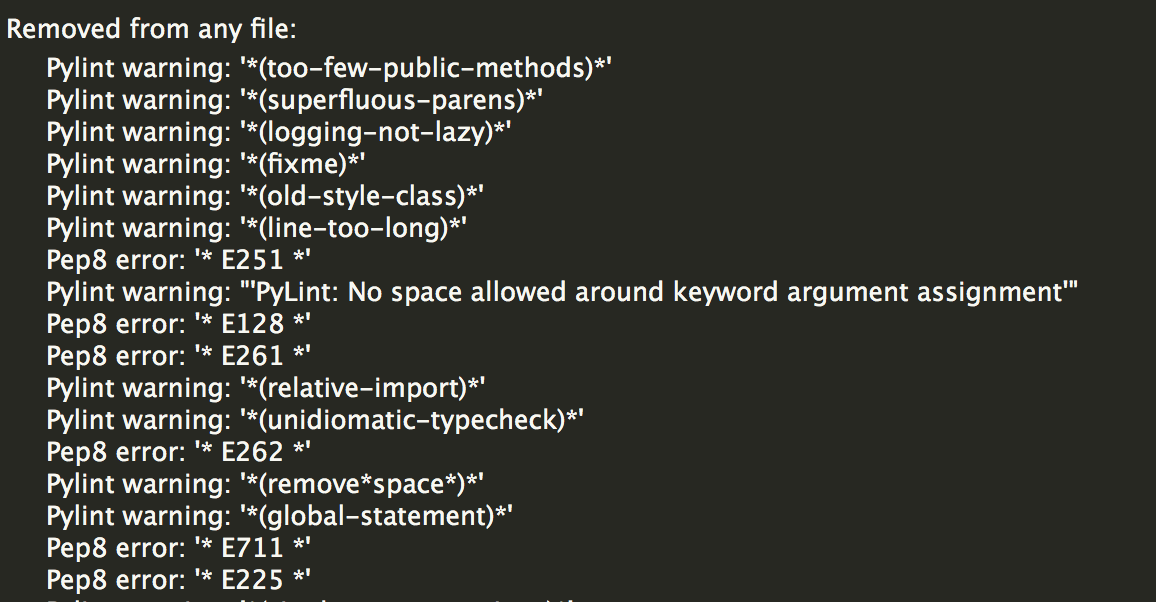
In this way, you should be able to drill down quickly to just the warnings that you are interested in.
You can export code warnings configurations or set the location Wing writes the configuration from the Options menu in the Code Warnings tool. This lets you share warnings configurations with other users or projects, for example by checking the configuration into revision control.
Recommendations
If you are required to write PEP 8 compatible code, enabling Wing's PEP 8 Reformatting feature may be preferable to using the pep8 code checker. This can reformat entire files or just the parts that you edit.
We encourage you to think carefully about how you use code checkers. While they do find errors early and can improve code quality, they may also lead to polishing that takes more time than it is worth. We've tried to design Wing's integration with external code checkers in a way that supports quick customization, so you can focus on the issues that you care about.
That's it for now! We'll be back next week with more Wing Tips for Wing Python IDE.
from Planet Python
via read more
metadsl: A Framework for Domain Specific Languages in Python
metadsl
: A Framework for Domain Specific Languages in Python¶
Hello, my name is Saul Shanabrook and for the past year or so I have been at Quansight exploring the array computing ecosystem. This started with working on the xnd project, a set of low level primitives to help build cross platform NumPy-like APIs, and then started exploring Lenore Mullin's work on a mathematics of arrays. After spending quite a bit of time working on an integrated solution built on these concepts, I decided to step back to try to generalize and simplify the core concepts. The trickiest part was not actually compiling mathematical descriptions of array operations in Python, but figuring out how to make it useful to existing users. To do this, we need to meet users where they are at, which is with the APIs they are already familiar with, like numpy
. The goal of metadsl
is to make it easier to tackle parts
from Planet SciPy
read more
Janusworx: A Week of Python
Ok. Time to be a bit honest. As you folks know, I have been trying to learn programming using Python since June 2017, when I joined the 10th cohort of DGPLUG’s Summer Training.
And time and again, I have failed.
Not just with programming, but with most other projects I tried to do.
At the end of my rope I decided to just quit everything and considered (very seriously) a return to my old stressful career, thinking maybe that is all there is for me.
Two people saved me.
The first one was Kushal Das.
The man was absolutely bull headed about me being in the right place and that if I could do this.
The other was my better half.
Everyday I count my blessings and am thankful that I that she chose to share her life with mine.
She patiently listens to my frustrated rants and then tells me to just dust myself up and do it again.
That failure is not the end of the world.
And then she told me to do my physio.
And that I really could do this.
Just because you failed doesn’t mean you can’t succeed.
We all fail. Mentally resilient people realize that its not failure that defines your identity but how you respond.
So towards the end of last year I decided to focus only on one or two things at once.
And at that time it meant my 12th exams.
I studied really hard for three months.
And I did not finish studying.
And I am pretty sure I am going to bomb my exam results.
Then why do I sound so chirpy?
Because I realised Kushal and Abby were right.
That I can in fact learn.
The past four months have been an exercise in frustration.
But I learnt something new everyday.
I could test myself on what I learnt and realise that I did in fact know stuff.
Which led me to my lightbulb moment.
That I cannot do all my learning like those montages they show in movies.
All my learning came from stretching just a tiny bit, every day.
I learnt the basics of Accounts, and lots of Maths.
The difficulty of a task is irrelevant, if it’s vital to your success.
— Ed Latimore
And now that exams are done, I decided to turn my attention back to programming.
And so I made a big ask of Kushal.1
I decided to go to Pune, and try to pick up the basics of programming in Python all over again.
And he graciously volunteered to mentor me for a week.
And here I am a week later, writing all sorts of tiny little programs that do whimsical things and bringing me joy.
I obviously have miles to go before I can even grasp at fluency.
But this time, I am filled with hope and a good measure of confidence. It’s been a little nerve wracking and there’s been tonnes of head scratching and back stretching.
Kushal has been extremely patient with me, guiding me these past few days, making sure I stretch just the right amount.
And for that I owe him a mountain of gratitude.
Thank you so much Kushal! I hope to pay it forward someday!
I go back home now, hoping to keep up the momentum with small incremental, regular periods of work.
I will log progress on the dtw blog where I can rant and rave to my hearts content.
My main focus will not be on results though.
Just to stretch myself everyday.
Improve myself just that little bit every day.
And then look back one day and be amazed at how far I’ve travelled.
The way you train reflects the way you fight.
People say I’m not going to train too hard, I’m going to do this in training, but when it’s time to fight I’m going to step up.There is no step up. You’re just going to do what you did every day.”
— Georges St. Pierre
from Planet Python
via read more
EuroPython Society: PyData EuroPython 2019
We are happy to announce a complete PyData track at EuroPython 2019:
![]()
PyData EuroPython 2019
The PyData track will be part of EuroPython 2019, so you won’t need to buy an extra ticket to attend.
The PyData track is run in cooperation with NumFocus.
There are two full tracks featuring more than 30 talks and 4 trainings:
- 4 trainings on Monday and Tuesday (July 8-9)
- 34 talks on Wednesday and Thursday (July 10-11)
- no PyData talks on Friday (July 12)
The full program is available on our PyData EuroPython 2019 page.
If you’d like to attend PyData EuroPython 2019, please register for EuroPython 2019 soon.
Dates and Venues
EuroPython 2019 will be held from July 8-14 2019 in Basel, Switzerland, at the Congress Center Basel (CCB) for the main conference days (Wed-Fri) and the FHNW Muttenz for the workshops/trainings/sprints days (Mon-Tue, Sat-Sun).
Please see our website for more information.
Enjoy,
–
EuroPython 2019 Team
https://ep2019.europython.eu/
https://www.europython-society.org/
from Planet Python
via read more
EuroPython: PyData EuroPython 2019
We are happy to announce a complete PyData track at EuroPython 2019:
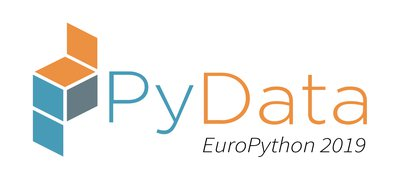
PyData EuroPython 2019
The PyData track will be part of EuroPython 2019, so you won’t need to buy an extra ticket to attend.
The PyData track is run in cooperation with NumFocus.
There are two full tracks featuring more than 30 talks and 4 trainings:
- 4 trainings on Monday and Tuesday (July 8-9)
- 34 talks on Wednesday and Thursday (July 10-11)
- no PyData talks on Friday (July 12)
The full program is available on our PyData EuroPython 2019 page.
If you’d like to attend PyData EuroPython 2019, please register for EuroPython 2019 soon.
Dates and Venues
EuroPython 2019 will be held from July 8-14 2019 in Basel, Switzerland, at the Congress Center Basel (CCB) for the main conference days (Wed-Fri) and the FHNW Muttenz for the workshops/trainings/sprints days (Mon-Tue, Sat-Sun).
Please see our website for more information.
Enjoy,
–
EuroPython 2019 Team
https://ep2019.europython.eu/
https://www.europython-society.org/
from Planet Python
via read more
Catalin George Festila: Python 3.7.3 : Testing firebase with Python 3.7.3 .
from Planet Python
via read more
Thursday, May 30, 2019
Wingware News: Wing Python IDE 7.0.3 - May 30, 2019
Wing 7.0.3 has been released. This is a minor release that includes the following fixes and improvements:
- Fix analysis when using Python 3 on a remote host
- Fix several cases where valid imports could not be resolved
- Improve default display scaling on Linux
- Correctly omit types and methods from debug data display
- Fix comparing remote files and buffer to remote disk
- Fix searching documentation in Search in Files
- Fix a number of other minor issues
You can obtain this release with Check for Updates in Wing 7's Help menu or download it now.
New in Wing 7
Wing 7 introduces an improved code warnings and code quality inspection system that includes built-in error detection and tight integration with Pylint, pep8, and mypy. This release also adds a new data frame and array viewer, a MATLAB keyboard personality, easy inline debug data display with Shift-Space, improved stack data display, support for PEP 3134 chained exceptions, callouts for search and other code navigation features, four new color palettes, improved bookmarking, a high-level configuration menu, magnified presentation mode, a new update manager, stepping over import internals, simplified remote agent installation, and much more.
For details see What's New in Wing 7
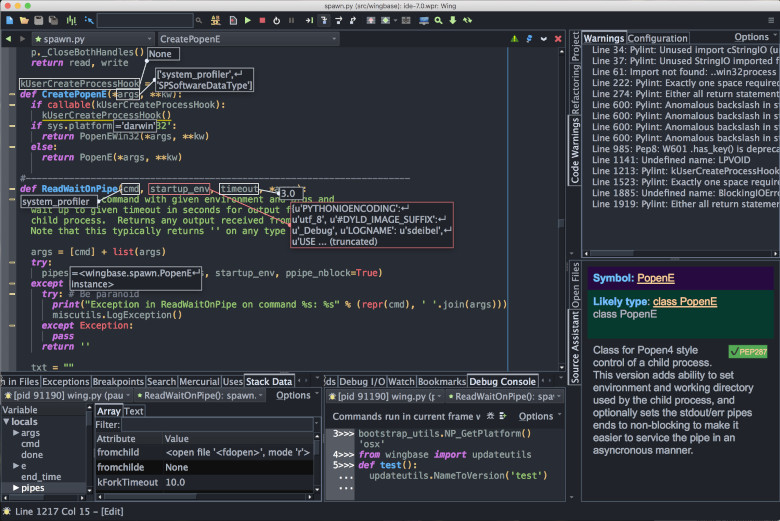
Try Wing 7 Now!
Wing 7 is a an exciting new step for Wingware's Python IDE product line. Find out how Wing 7 can turbocharge your Python development by trying it today.
Download Wing 7.0.3: Wing Pro | Wing Personal | Wing 101 | Compare Products
Wing 7 installs side by side with earlier versions of Wing, so there is no need to remove old versions in order to try it. Wing 7 will read and convert your old preferences, settings, and projects. Projects should be saved to a new name since earlier versions of Wing cannot read Wing 7 projects.
See Upgrading for details and Migrating from Older Versions for a list of compatibility notes.
from Planet Python
via read more
Learn PyQt: MacOS Mojave Dark Mode Support in PyQt 5.12.2
MacOS Mojave, released in September of last year, introduced a user-toggleable "Dark Mode". This enables a system-wide dark colour scheme, intended to make things easier on your eyes at night, or less distracting when working with content. The mode was supported by all built-in Mac apps on release, but 3rd party applications (including those developed with PyQt) were left looking a bit out of place.
The support for Dark Mode in Qt was targeted for 5.12 which landed in December 2018, with the first Python support in PyQt 5.12 released February 2019.
Early implementations had a few issues (see below) but as of PyQt 5.12.2 it's looking great. The pictures below show the same example app (just a random assortment of widgets) under Dark Mode and (default) Light Mode on MacOS Mojave.
pip3 install pyqt5==5.12.2
If you're on PyQt 5.12.2 or over Dark Mode will work out of the box.


Issues on earlier versions
While PyQt 5.2.12 applications look great in Dark Mode, earlier versions have had a few issues. Below are a few screenshots of the same example app taken across earlier releases. If you're releasing your PyQt 5 apps to MacOS you might want to take a look at how it handles.
Downgrading to PyQt 5.12 produces the UI below — more or less fine, but with a missing colour on the spin-wheel nubbin. It doesn't effect the functioning of the app, just looks a bit less nice.
pip3 install pyqt5==5.12

Downgrading further to PyQt 5.10 produces this disaster. Strangely the wheel is now shaded properly, but the text is white-on-white.
pip3 install pyqt5==5.10

Weirdly, although PyQt 5.11 looks just as bad, 5.9 looks slightly better (as in potentially usable).
pip3 install pyqt5==5.9

The bottom line is: if you're targeting MacOS with your applications and using < PyQt5.12.2 then now would be a very good time to upgrade and ensure your app looks as great as it can under Mojave Dark Mode.
from Planet Python
via read more
Use two-factor auth to improve your PyPI account's security
Starting today, the canonical Python Package Index at PyPI.org and the test site at test.pypi.org offer 2FA for all users. We encourage project maintainers and owners to log in and go to their Account Settings to add a second factor. This will help improve the security of their PyPI user accounts, and thus reduce the risk of vandals, spammers, and thieves gaining account access.
PyPI's maintainers tested this new feature throughout May and fixed several resulting bug reports; regardless, you might find a new issue. If you find any potential security vulnerabilities, please follow our published security policy. (Please don't report security issues in Warehouse via GitHub, IRC, or mailing lists. Instead, please directly email one or more of our maintainers.) If you find an issue that is not a security vulnerability, please report it via GitHub.
PyPI currently supports a single 2FA method: generating a code through a Time-based One-time Password (TOTP) application. After you set up 2FA on your PyPI account, then you must provide a TOTP (along with your username and password) to log in. Therefore, to use 2FA on PyPI, you'll need to provision an application (usually a mobile phone app) in order to generate authentication codes; see our FAQ for suggestions and pointers.
You'll need to verify your primary email address on your Test PyPI and/or PyPI accounts before setting up 2FA. You can also do that in your Account Settings.
Currently, only TOTP is supported as a 2FA method. Also, 2FA only affects login via the website which safeguards against malicious changes to project ownership, deletion of old releases, and account take overs. Package uploads will continue to work without 2FA codes being provided.
But we're not done! We're currently working on WebAuthn-based multi-factor authentication, which will let you use, for instance, Yubikeys for your second factor. Then we'll add API keys for package upload, then an advanced audit trail of sensitive user actions. More details are in our progress reports.
Thanks to the Open Technology Fund for funding this work. And please sign up for the PyPI Announcement Mailing List for future updates.
from Python Insider
read more
Dataquest: Python For Loops: A Tutorial
Learn how to master Python for loops and statements like break and continue to iterate through lists and clean and analyze large data sets quickly.
The post Python For Loops: A Tutorial appeared first on Dataquest.
from Planet Python
via read more
Python Insider: Use two-factor auth to improve your PyPI account's security
Starting today, the canonical Python Package Index at PyPI.org and the test site at test.pypi.org offer 2FA for all users. We encourage project maintainers and owners to log in and go to their Account Settings to add a second factor. This will help improve the security of their PyPI user accounts, and thus reduce the risk of vandals, spammers, and thieves gaining account access.
PyPI's maintainers tested this new feature throughout May and fixed several resulting bug reports; regardless, you might find a new issue. If you find any potential security vulnerabilities, please follow our published security policy. (Please don't report security issues in Warehouse via GitHub, IRC, or mailing lists. Instead, please directly email one or more of our maintainers.) If you find an issue that is not a security vulnerability, please report it via GitHub.
PyPI currently supports a single 2FA method: generating a code through a Time-based One-time Password (TOTP) application. After you set up 2FA on your PyPI account, then you must provide a TOTP (along with your username and password) to log in. Therefore, to use 2FA on PyPI, you'll need to provision an application (usually a mobile phone app) in order to generate authentication codes; see our FAQ for suggestions and pointers.
You'll need to verify your primary email address on your Test PyPI and/or PyPI accounts before setting up 2FA. You can also do that in your Account Settings.
Currently, only TOTP is supported as a 2FA method. Also, 2FA only affects login via the website which safeguards against malicious changes to project ownership, deletion of old releases, and account take overs. Package uploads will continue to work without 2FA codes being provided.
But we're not done! We're currently working on WebAuthn-based multi-factor authentication, which will let you use, for instance, Yubikeys for your second factor. Then we'll add API keys for package upload, then an advanced audit trail of sensitive user actions. More details are in our progress reports.
Thanks to the Open Technology Fund for funding this work. And please sign up for the PyPI Announcement Mailing List for future updates.
from Planet Python
via read more
Python Software Foundation: Use two-factor auth to improve your PyPI account's security
Starting today, the canonical Python Package Index at PyPI.org and the test site at test.pypi.org offer 2FA for all users. We encourage project maintainers and owners to log in and go to their Account Settings to add a second factor. This will help improve the security of their PyPI user accounts, and thus reduce the risk of vandals, spammers, and thieves gaining account access.
PyPI's maintainers tested this new feature throughout May and fixed several resulting bug reports; regardless, you might find a new issue. If you find any potential security vulnerabilities, please follow our published security policy. (Please don't report security issues in Warehouse via GitHub, IRC, or mailing lists. Instead, please directly email one or more of our maintainers.) If you find an issue that is not a security vulnerability, please report it via GitHub.
PyPI currently supports a single 2FA method: generating a code through a Time-based One-time Password (TOTP) application. After you set up 2FA on your PyPI account, then you must provide a TOTP (along with your username and password) to log in. Therefore, to use 2FA on PyPI, you'll need to provision an application (usually a mobile phone app) in order to generate authentication codes; see our FAQ for suggestions and pointers.
You'll need to verify your primary email address on your Test PyPI and/or PyPI accounts before setting up 2FA. You can also do that in your Account Settings.
Currently, only TOTP is supported as a 2FA method. Also, 2FA only affects login via the website which safeguards against malicious changes to project ownership, deletion of old releases, and account take overs. Package uploads will continue to work without 2FA codes being provided.
But we're not done! We're currently working on WebAuthn-based multi-factor authentication, which will let you use, for instance, Yubikeys for your second factor. Then we'll add API keys for package upload, then an advanced audit trail of sensitive user actions. More details are in our progress reports.
Thanks to the Open Technology Fund for funding this work. And please sign up for the PyPI Announcement Mailing List for future updates.
from Planet Python
via read more
Use two-factor auth to improve your PyPI account's security
Starting today, the canonical Python Package Index at PyPI.org and the test site at test.pypi.org offer 2FA for all users. We encourage project maintainers and owners to log in and go to their Account Settings to add a second factor. This will help improve the security of their PyPI user accounts, and thus reduce the risk of vandals, spammers, and thieves gaining account access.
PyPI's maintainers tested this new feature throughout May and fixed several resulting bug reports; regardless, you might find a new issue. If you find any potential security vulnerabilities, please follow our published security policy. (Please don't report security issues in Warehouse via GitHub, IRC, or mailing lists. Instead, please directly email one or more of our maintainers.) If you find an issue that is not a security vulnerability, please report it via GitHub.
PyPI currently supports a single 2FA method: generating a code through a Time-based One-time Password (TOTP) application. After you set up 2FA on your PyPI account, then you must provide a TOTP (along with your username and password) to log in. Therefore, to use 2FA on PyPI, you'll need to provision an application (usually a mobile phone app) in order to generate authentication codes; see our FAQ for suggestions and pointers.
You'll need to verify your primary email address on your Test PyPI and/or PyPI accounts before setting up 2FA. You can also do that in your Account Settings.
Currently, only TOTP is supported as a 2FA method. Also, 2FA only affects login via the website which safeguards against malicious changes to project ownership, deletion of old releases, and account take overs. Package uploads will continue to work without 2FA codes being provided.
But we're not done! We're currently working on WebAuthn-based multi-factor authentication, which will let you use, for instance, Yubikeys for your second factor. Then we'll add API keys for package upload, then an advanced audit trail of sensitive user actions. More details are in our progress reports.
Thanks to the Open Technology Fund for funding this work. And please sign up for the PyPI Announcement Mailing List for future updates.
from Python Software Foundation News
via read more
Catalin George Festila: Python 3.7.3 : Using the win32com - part 007.
from Planet Python
via read more
Catalin George Festila: Python 3.7.3 : How fast are Data Classes.
from Planet Python
via read more
Codementor: Powering up python as a data analysis platform
from Planet Python
via read more
Wednesday, May 29, 2019
Python Software Foundation: PyPI Security and Accessibility Q1 2019 Request for Proposals Update
The initial deadline for our RFP was December 14th. We had hoped to begin work with the selected proposers in January 2019, but ultimately fell short of the ability to do so.
What’s holding us back
After the deadline passed there were no proposals submitted for Milestone 2. This leaves us in a position where the project cannot proceed as planned without reassessing the RFP process and extending the deadline.
How we’ll move forward
The RFP document has been updated based on feedback received from those who took part in the initial period to allow for additional flexibility on proposal parameters.
The RFP contained two milestones that could be proposed for independently or as a pair. In responses to our RFP the security development milestone (Milestone 1) received more attention than the accessibility and internationalization milestone (Milestone 2).
Given that we will at least need to extend the RFP period to obtain proposals for Milestone 2, we plan to continue to accept proposals for both. This offers us the best chance to select a proposal that will best utilize the available funds.
Our new deadline for responses will be the end of the day January 31st, 2019 AoE. You can read the full Request for Proposals document here.
If you have any questions, concerns, or feedback about the RFP please contact the Python Software Foundation Director of Infrastructure, Ernest W. Durbin III. Proposers may also discuss the RFP in our community discussion forum at discuss.python.org.
from Planet Python
via read more
Python Software Foundation: 2018 in review!
Happy New Year from the PSF! We’d like to highlight some of our activities from 2018 and update the community on the initiatives we are working on.
PyCon 2018
Registration is now open for PyCon 2019: https://pycon.blogspot.com/2018/11/pycon-2019-registration-is-open.html .
Community Support
To help with transparency, the PSF launched its first newsletter in December! If you’d like to receive our next edition, subscribe here: https://www.python.org/psf/newsletter/. You can read our first edition here: https://mailchi.mp/53049c7e2d8b/python-software-foundation-q4-newsletter
Python Package Index
Grants
Here is a chart showing the global grant distribution in 2018:
PSF Staff
In September, the PSF hired Jackie Augustine as Event Manager. Jackie will be working with the team on all facets of PyCon and managing several community resources for regional conferences.
It is with great pleasure that we announce that Ewa Jodlowska will be the PSF's first Executive Director, starting January 1, 2019. Given her years of dedicated service to the PSF from event manager to her current position as Director of Operations, we can think of no one more qualified to fill this role as the PSF continues to grow and develop.
Community Recognition
Community Service Awards
Chukwudi Nwachukwu was recognized for his contribution to spreading the growth of Python to the Nigerian community and his dedication and research to the PSF grants work group.Mario Corchero was awarded a CSA for his leadership of the organization of PyConES, PyLondinium, and the PyCon Charlas track in 2018. His work has been instrumental in promoting the use of Python and fostering Python communities in Spain, Latin America, and the UK.
We also honored our Job Board volunteers: Jon Clements, Melanie Jutras, Rhys Yorke, Martijn Pieters, Patrice Neff, and Marc-Andre Lemburg, who have spent many hours reviewing and managing the hundreds of job postings submitted on an annual basis
Mariatta Wijaya was an awardee for her contributions to CPython, her efforts to improve the workflow of the Python core team, and her work to increase diversity in our community. In addition, her work as co-chair of PyCascades helps spread the growth of Python
Alex Gaynor received an award for his contributions to the Python and Django Communities and the Python Software Foundation. Alex previously served as a PSF Director in 2015-2016. He currently serves as an Infrastructure Staff member and contributes to legacy PyPI and the next generation warehouse and has helped legacy warehouse in security (disabling unsupported OpenID) and cutting bandwidth costs by compressing 404 images.
2018 Distinguished Service Award
The 2018 Distinguished Service Award was presented to Marc-Andre Lemburg for his significant contributions to Python as a core developer, EuroPython chair, PSF board member, and board member of the EuroPython Society.2018 Frank Willison Memorial Award
The Frank Willison Memorial Award for Contributions to the Python Community was awarded to Audrey Roy Greenfeld and Daniel Roy Greenfeld for their contributions to the development of Python and the global Python community through their speaking, teaching, and writing.Donations and Sponsorships
We welcome your thoughts on how you’d like to see our Foundation involved in Python’s ecosystem and are always interested in hearing from you. Email us!
We wish you a very successful 2019!
Ewa Jodlowska
Executive Director
Betsy Waliszewski
Sponsor Coordinator
from Planet Python
via read more
Python Software Foundation: Upcoming PyPI Improvements for 2019
Facebook Gift
Open Technology Fund
Get Involved
from Planet Python
via read more
TestDriven.io: Working with Static and Media Files in Django
This article looks at how to work with static and media files in a Django project, locally and in production. from Planet Python via read...
-
Podcasts are a great way to immerse yourself in an industry, especially when it comes to data science. The field moves extremely quickly, an...
-
In an earlier tutorial we've already covered how to open dialog windows. These are special windows which (by default) grab the focus o...
-
If you are already developing Python GUI apps with PySide2, you might be asking yourself whether it's time to upgrade to PySide6 and use...