Building small projects, like a text-based user interface (TUI) dice-rolling application, will help you level up your Python programming skills. You’ll learn how to gather and validate the user’s input, import code from modules and packages, write functions, use for
loops and conditionals, and neatly display output by using strings and the print()
function.
In this project, you’ll code an application that simulates dice-rolling events. To do so, you’ll use Python’s random
module.
In this tutorial, you’ll learn how to:
- Use
random.randint()
to simulate dice-rolling events - Ask for the user’s input using the built-in
input()
function - Parse and validate the user’s input
- Manipulate strings using methods, such as
.center()
and.join()
You’ll also learn the basics of how to structure, organize, document, and run your Python programs and scripts.
Click the link below to download the entire code for this dice-rolling application and follow along while you build the project yourself:
Get Source Code: Click here to get the source code you’ll use to build your Python dice-rolling app.
Demo
In this step-by-step project, you’ll build an application that runs dice-rolling simulations. The app will be able to roll up to six dice, with each die having six faces. After every roll, the application will generate an ASCII diagram of dice faces and display it on the screen. The following video demonstrates how the app works:
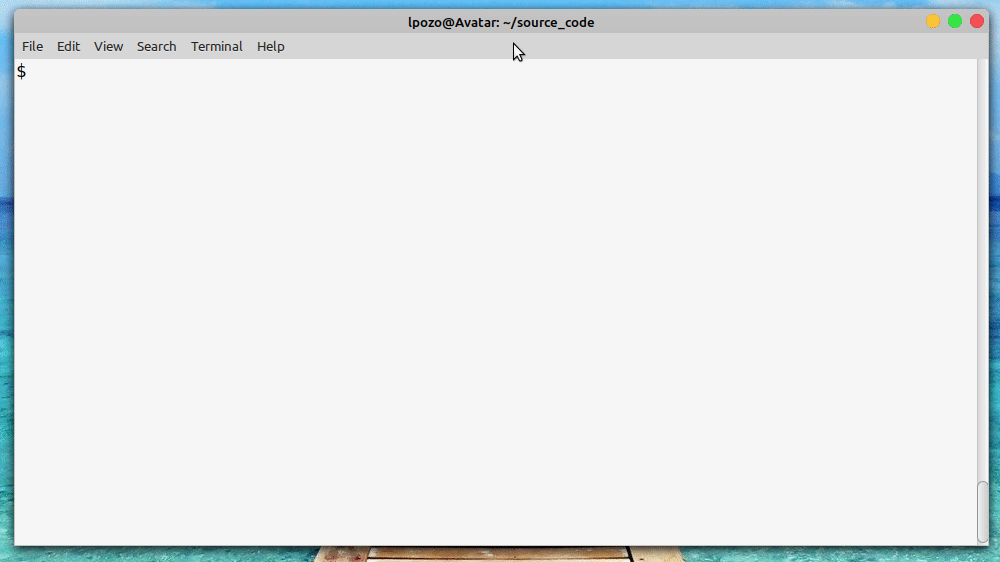
When you run your dice-rolling simulator app, you get a prompt asking for the number of dice you want to roll. Once you provide a valid integer from 1 to 6, inclusive, then the application simulates the rolling event and displays a diagram of dice faces on the screen.
Project Overview
Your dice-rolling simulator app will have a minimal yet user-friendly text-based user interface (TUI), which will allow you to specify the number of six-sided dice that you’d like to roll. You’ll use this TUI to roll the dice at home without having to fly to Las Vegas.
Here’s a description of how the app will work internally:
Tasks to Run | Tools to Use | Code to Write |
---|---|---|
Prompt the user to choose how many six-sided dice to roll, then read the user’s input | Python’s built-in input() function |
A call to input() with appropriate arguments |
Parse and validate the user’s input | String methods, comparison operators, and conditional statements | A user-defined function called parse_input() |
Run the dice-rolling simulation | Python’s random module, specifically the randint() function |
A user-defined function called roll_dice() |
Generate an ASCII diagram with the resulting dice faces | Loops, list.append() , and str.join() |
A user-defined function called generate_dice_faces_diagram() |
Display the diagram of dice faces on the screen | Python’s built-in print() function |
A call to print() with appropriate arguments |
Keeping these internal workings in mind, you’ll code three custom functions to provide the app’s main features and functionalities. These functions will define your code’s public API, which you’ll call to bring the app to life.
To organize the code of your dice-rolling simulator project, you’ll create a single file called dice.py
in a directory of your choice in your file system. Go ahead and create the file to get started!
Prerequisites
You should be comfortable with the following concepts and skills before you start building this dice-rolling simulation project:
- Ways to run scripts in Python
- Python’s
import
mechanism - The basics of Python data types, mainly strings and integer numbers
- Basic data structures, especially lists
- Python variables and constants
- Python comparison operators
- Boolean values and logical expressions
- Conditional statements
- Python
for
loops - The basics of input, output, and string formatting in Python
If you don’t have all of the prerequisite knowledge before starting this coding adventure, then that’s okay! You might learn more by going ahead and getting started! You can always stop and review the resources linked here if you get stuck.
Step 1: Code the TUI of Your Python Dice-Rolling App
In this step, you’ll write the required code to ask for the user’s input of how many dice they want to roll in the simulation. You’ll also code a Python function that takes the user’s input, validates it, and returns it as an integer number if the validation was successful. Otherwise, the function will ask for the user’s input again.
To download the code for this step, click the following link and navigate to the source_code_step_1/
folder:
Get Source Code: Click here to get the source code you’ll use to build your Python dice-rolling app.
Read the full article at https://realpython.com/python-dice-roll/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
from Real Python
read more
No comments:
Post a Comment