The fractions
module in Python is arguably one of the most underused elements of the standard library. Even though it may not be well-known, it’s a useful tool to have under your belt because it can help address the shortcomings of floating-point arithmetic in binary. That’s essential if you plan to work with financial data or if you require infinite precision for your calculations.
Towards the end of this tutorial, you’ll see a few hands-on examples where fractions are the most suitable and elegant choice. You’ll also learn about their weaknesses and how to make the best use of them along the way.
In this tutorial, you’ll learn how to:
- Convert between decimal and fractional notation
- Perform rational number arithmetic
- Approximate irrational numbers
- Represent fractions exactly with infinite precision
- Know when to choose
Fraction
overDecimal
orfloat
The majority of this tutorial goes over the fractions
module, which in itself doesn’t require in-depth Python knowledge other than an understanding of its numeric types. However, you’ll be in a good place to work through all the code examples that follow if you’re familiar with more advanced concepts such as Python’s built-in collections
module, itertools
module, and generators. You should already be comfortable with these topics if you want to make the most out of this tutorial.
Free Bonus: 5 Thoughts On Python Mastery, a free course for Python developers that shows you the roadmap and the mindset you’ll need to take your Python skills to the next level.
Decimal vs Fractional Notation
Let’s take a walk down memory lane to bring back your school knowledge of numbers and avoid possible confusion. There are four concepts at play here:
- Types of numbers in mathematics
- Numeral systems
- Notations of numbers
- Numeric data types in Python
You’ll get a quick overview of each of these now to better understand the purpose of the Fraction
data type in Python.
Classification of Numbers
If you don’t remember the classification of numbers, here’s a quick refresher:
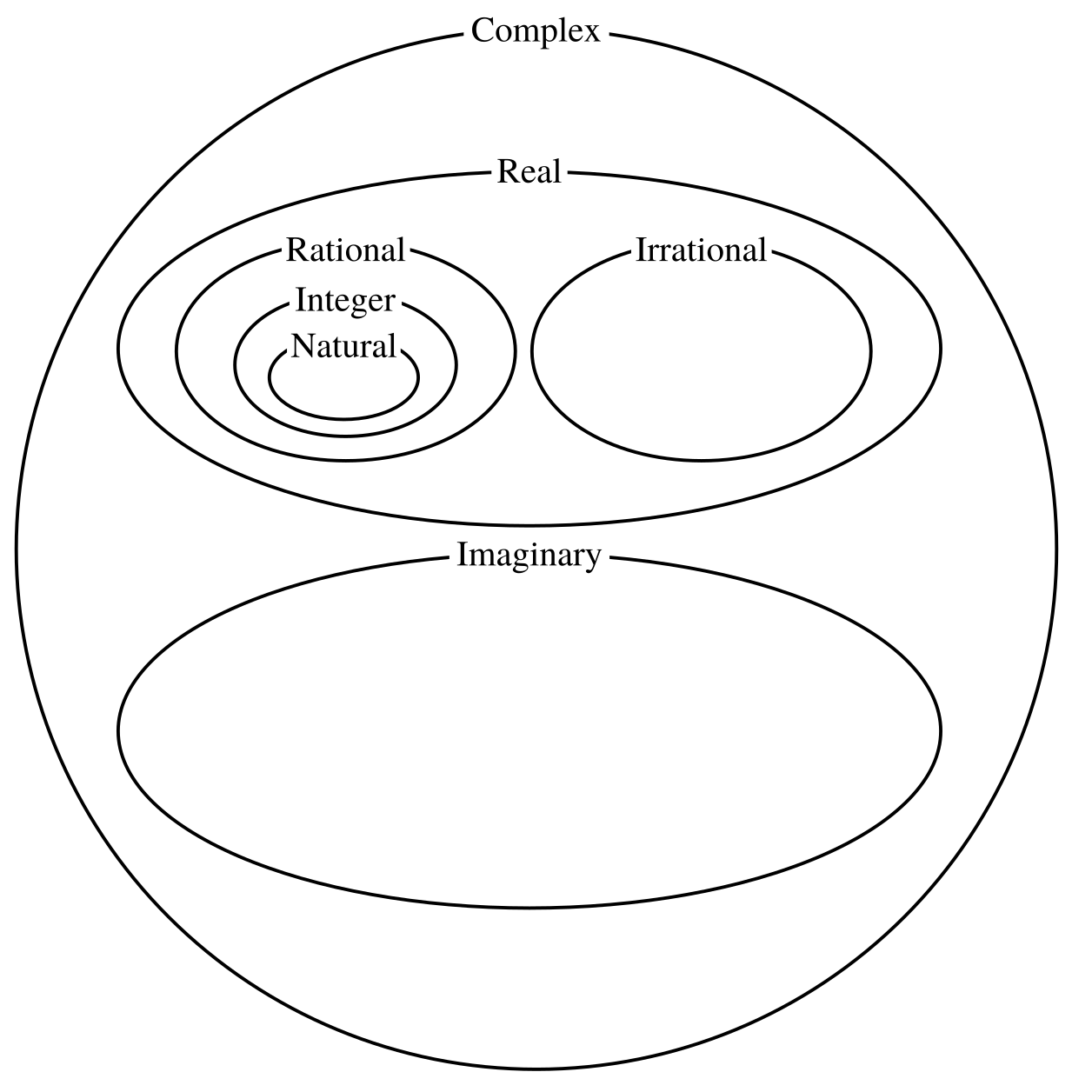
There are many more types of numbers in mathematics, but these are the most relevant in day-to-day life. At the very top, you’ll find complex numbers that include imaginary and real numbers. Real numbers are, in turn, comprised of rational and irrational numbers. Finally, rational numbers contain integers and natural numbers.
Numeral Systems and Notations
There have been various systems of expressing numbers visually over the centuries. Today, most people use a positional numeral system based on Hindu-Arabic symbols. You can choose any base or radix for such a system. However, while people prefer the decimal system (base-10), computers work best in the binary system (base-2).
Within the decimal system itself, you can represent some numbers using alternative notations:
- Decimal: 0.75
- Fractional: ¾
Neither of these is better or more precise than the other. Expressing a number in decimal notation is perhaps more intuitive because it resembles a percentage. Comparing decimals is also more straightforward since they already have a common denominator—the base of the system. Finally, decimal numbers can communicate precision by keeping the trailing and leading zeros.
On the other hand, fractions are more convenient in performing symbolic algebra by hand, which is why they’re mainly used in school. But can you recall the last time you used fractions? If you can’t, then that’s because decimal notation is central in calculators and computers nowadays.
The fractional notation is typically associated with rational numbers only. After all, the very definition of a rational number states that you can express it as a quotient, or a fraction, of two integers as long as the denominator is nonzero. However, that’s not the whole story when you factor in infinite continued fractions that can approximate irrational numbers:
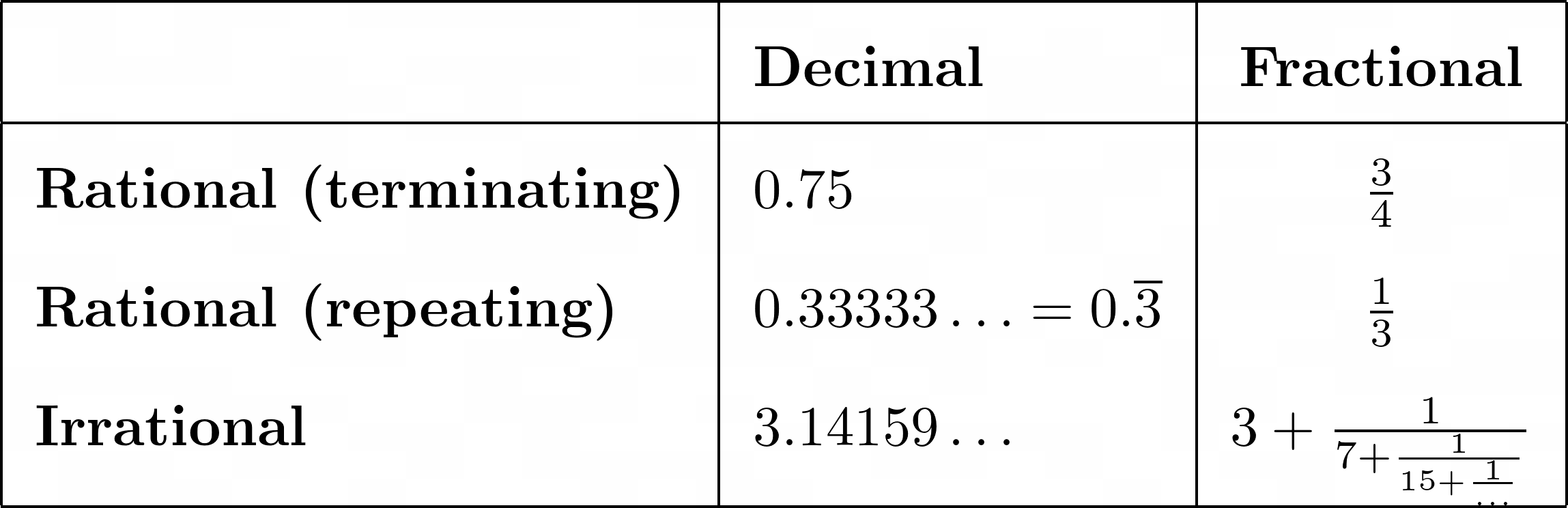
Irrational numbers always have a non-terminating and non-repeating decimal expansion. For example, the decimal expansion of pi (π) never runs out of digits that seem to have a random distribution. If you were to plot their histogram, then each digit would have a roughly similar frequency.
On the other hand, most rational numbers have a terminating decimal expansion. However, some can have an infinite recurring decimal expansion with one or more digits repeated over a period. The repeated digits are commonly denoted with an ellipsis (0.33333…) in the decimal notation. Regardless of their decimal expansion, rational numbers such as the number representing one-third always look elegant and compact in the fractional notation.
Numeric Data Types in Python
Read the full article at https://realpython.com/python-fractions/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
from Real Python
read more
No comments:
Post a Comment