Are you diving deeper into Python lists and wanting to learn about different ways to reverse them? If so, then this tutorial is for you. Here, you’ll learn about a few Python tools and techniques that are handy when it comes to reversing lists or manipulating them in reverse order. This knowledge will complement and improve your list-related skills and make you more proficient with them.
In this tutorial, you’ll learn how to:
- Reverse existing lists in place using
.reverse()
and other techniques - Create reversed copies of existing lists using
reversed()
and slicing - Use iteration, comprehensions, and recursion to create reversed lists
- Iterate over your lists in reverse order
- Sort your lists in reverse order using
.sort()
andsorted()
To get the most out of this tutorial, it would be helpful to know the basics of iterables, for
loops, lists, list comprehensions, generator expressions, and recursion.
Free Bonus: Click here to get a Python Cheat Sheet and learn the basics of Python 3, like working with data types, dictionaries, lists, and Python functions.
Reversing Python Lists
Sometimes you need to process Python lists starting from the last element down to the first—in other words, in reverse order. In general, there are two main challenges related to working with lists in reverse:
- Reversing a list in place
- Creating reversed copies of an existing list
To meet the first challenge, you can use either .reverse()
or a loop that swaps items by index. For the second, you can use reversed()
or a slicing operation. In the next sections, you’ll learn about different ways to accomplish both in your code.
Reversing Lists in Place
Like other mutable sequence types, Python lists implement .reverse()
. This method reverses the underlying list in place for memory efficiency when you’re reversing large list objects. Here’s how you can use .reverse()
:
>>> digits = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> digits.reverse()
>>> digits
[9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
When you call .reverse()
on an existing list, the method reverses it in place. This way, when you access the list again, you get it in reverse order. Note that .reverse()
doesn’t return a new list but None
:
>>> digits = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> reversed_digits = digits.reverse()
>>> reversed_digits is None
True
Trying to assign the return value of .reverse()
to a variable is a common mistake related to using this method. The intent of returning None
is to remind its users that .reverse()
operates by side effect, changing the underlying list.
Note: Most of the examples in this tutorial use a list of numbers as input. However, the same tools and techniques apply to lists of any type of Python objects, such as lists of strings.
Okay! That was quick and straightforward! Now, how can you reverse a list in place by hand? A common technique is to loop through the first half of it while swapping each element with its mirror counterpart on the second half of the list.
Python provides zero-based positive indices to walk sequences from left to right. It also allows you to navigate sequences from right to left using negative indices:
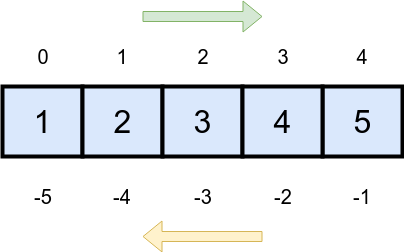
This diagram shows that you can access the first element of the list (or sequence) using either 0
or -5
with the indexing operator, like in sequence[0]
and sequence[-5]
, respectively. You can use this Python feature to reverse the underlying sequence in place.
For example, to reverse the list represented in the diagram, you can loop over the first half of the list and swap the element at index 0
with its mirror at index -1
in the first iteration. Then you can switch the element at index 1
with its mirror at index -2
and so on until you get the list reversed.
Here’s a representation of the whole process:
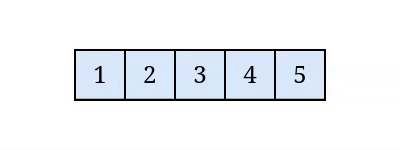
To translate this process into code, you can use a for
loop with a range
object over the first half of the list, which you can get with len(digits) // 2
. Then you can use a parallel assignment statement to swap the elements, like this:
>>> digits = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> for i in range(len(digits) // 2):
... digits[i], digits[-1 - i] = digits[-1 - i], digits[i]
...
>>> digits
[9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
Read the full article at https://realpython.com/python-reverse-list/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
from Planet Python
via read more
No comments:
Post a Comment