Adding items to a list is a fairly common task in Python, so the language provides a bunch of methods and operators that can help you out with this operation. One of those methods is .append()
. With .append()
, you can add items to the end of an existing list object. You can also use .append()
in a for
loop to populate lists programmatically.
In this tutorial, you’ll learn how to:
- Work with
.append()
- Populate lists using
.append()
and afor
loop - Replace
.append()
with list comprehensions - Work with
.append()
inarray.array()
andcollections.deque()
You’ll also code some examples of how to use .append()
in practice. With this knowledge, you’ll be able to effectively use .append()
in your programs.
Free Download: Get a sample chapter from Python Basics: A Practical Introduction to Python 3 to see how you can go from beginner to intermediate in Python with a complete curriculum, up-to-date for Python 3.8.
Adding Items to a List With Python’s .append()
Python’s .append()
takes an object as an argument and adds it to the end of an existing list, right after its last element:
>>> numbers = [1, 2, 3]
>>> numbers.append(4)
>>> numbers
[1, 2, 3, 4]
Every time you call .append()
on an existing list, the method adds a new item to the end, or right side, of the list. The following diagram illustrates the process:
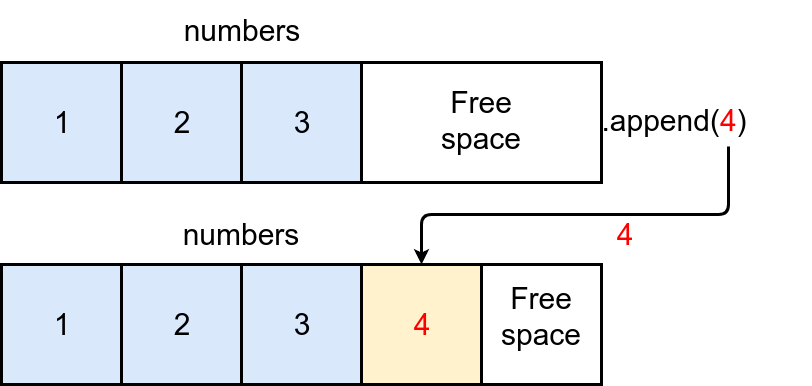
Python lists reserve extra space for new items at the end of the list. A call to .append()
will place new items in the available space.
In practice, you can use .append()
to add any kind of object to a given list:
>>> mixed = [1, 2]
>>> mixed.append(3)
>>> mixed
[1, 2, 3]
>>> mixed.append("four")
>>> mixed
[1, 2, 3, 'four']
>>> mixed.append(5.0)
>>> mixed
[1, 2, 3, 'four', 5.0]
Lists are sequences that can hold different data types and Python objects, so you can use .append()
to add any object to a given list. In this example, you first add an integer number, then a string, and finally a floating-point number. However, you can also add another list, a dictionary, a tuple, a user-defined object, and so on.
Using .append()
is equivalent to the following operation:
>>> numbers = [1, 2, 3]
>>> # Equivalent to numbers.append(4)
>>> numbers[len(numbers):] = [4]
>>> numbers
[1, 2, 3, 4]
In the highlighted line, you perform two operations at the same time:
- You take a slice from
numbers
using the expressionnumbers[len(numbers):]
. - You assign an iterable to that slice.
The slicing operation takes the space after the last item in numbers
. Meanwhile, the assignment operation unpacks the items in the list to the right of the assignment operator and adds them to numbers
. However, there’s an important difference between using this kind of assignment and using .append()
. With the assignment, you can add several items to the end of your list at once:
>>> numbers = [1, 2, 3]
>>> numbers[len(numbers):] = [4, 5, 6]
>>> numbers
[1, 2, 3, 4, 5, 6]
In this example, the highlighted line takes a slice from the end of numbers
, unpacks the items in the list on the right side, and adds them to the slice as individual items.
.append()
Adds a Single Item
With .append()
, you can add a number, list, tuple, dictionary, user-defined object, or any other object to an existing list. However, you need to keep in mind that .append()
adds only a single item or object at a time:
>>> x = [1, 2, 3, 4]
>>> y = (5, 6)
>>> x.append(y)
>>> x
[1, 2, 3, 4, (5, 6)]
What happens here is that .append()
adds the tuple object y
to the end of your target list, x
. What if you want to add each item in y
to the end of x
as an individual item and get [1, 2, 3, 4, 5, 6]
? In that case, you can use .extend()
:
Read the full article at https://realpython.com/python-append/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
from Real Python
read more
No comments:
Post a Comment