How to Use Python’s Print() Without Adding an Extra New Line
Here’s how you can avoid superfluous newlines when using the print function or statement in Python 2.x and 3.x
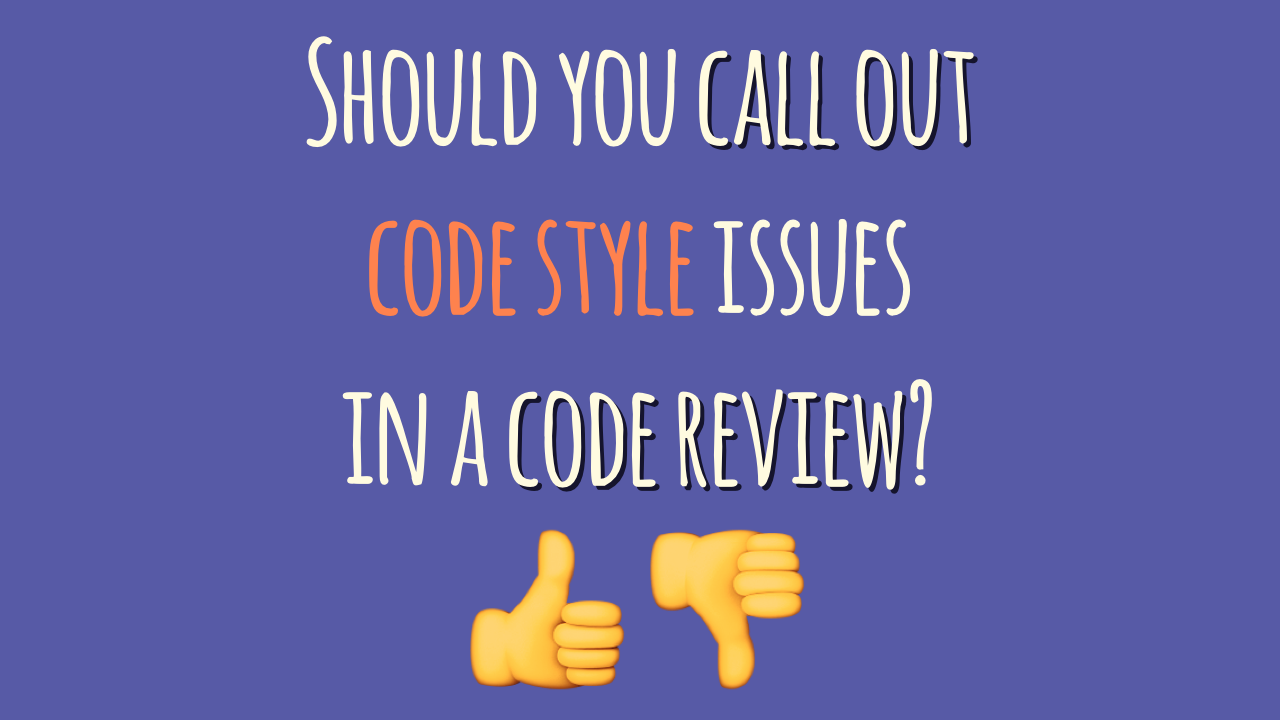
Let’s say you’re working on a Python script that prints out progress updates to the console while it does its work in order to keep the user informed.
Maybe you’ll want the output to look something like this:
Processing files ................ Complete
With another .
dot printed every time a file has been processed. Now how would you implement this in Python?
If you try the following, you’ll get a botched output:
print('Processing files') for i in range(5): print('.') print('Complete')
Processing files . . . . . Complete
As you can see, the .
dot characters are printed on a new line each instead of one long consecutive line. How do you get Python to print them all on one line without newlines after each character?
Python 2.x and 3.x – sys.stdout.write()
import sys print('Processing files') for i in range(5): sys.stdout.write('.') print('Complete')
If you want to use sys.stdout.write
for printing the other messages as well, you’ll need to make sure to add the newlines manually yourself. You can do this by adding a \n
linefeed special character to your message strings, like so:
import sys sys.stdout.write('Processing files\n') for i in range(5): sys.stdout.write('.') sys.stdout.write('Complete\n')
Depending on your terminal setup you might not see those dots printed immediately if you’re working with a bit of a delay between printing each characters. If this happens you can call the sys.stdout.flush()
function to ensure any pending characters are written to the console.
Python 3.x – print()
function
Python 2.x – print
statement
Let’s say you wanted to print the following string of numbers in Python:
1, 2, 3, 4, 5
>>> for i in range(5): ... print(i + ', ') 0, 1, 2, 3, 4,
132 down vote accepted In Python 3.x, you can use the end argument to the print() function to prevent a newline character from being printed:
print(“Nope, that is not a two. That is a”, end=”“) In Python 2.x, you can use a trailing comma:
print “this should be”, print “on the same line” You don’t need this to simply print a variable, though:
print “Nope, that is not a two. That is a”, x Note that the trailing comma still results in a space being printed at the end of the line, i.e. it’s equivalent to using end=” ” in Python 3. To suppress the space character as well, you can either use
from future import print_function to get access to the Python 3 print function or use sys.stdout.write().
[ Improve Your Python with Dan's 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
from Planet Python
via read more
No comments:
Post a Comment