Hello programmers, today, we will learn about the implementation of Matplotlib tables in Python. The matplotlib.pyplot.table() method is used to create or add a table to axes in python programs. It generates a table used as an extension to a stacked bar chart. Before we move on with various examples and formatting of tables, let me just brief you about the syntax and return type of the Matplotlib table function.
Syntax of Matplotlib Table:
matplotlib.pyplot.table
(cellText=None, cellColours=None, cellLoc='right', colWidths=None, rowLabels=None, rowColours=None, rowLoc='left', colLabels=None, colColours=None, colLoc='center', loc='bottom', bbox=None, edges='closed', **kwargs)
Specifying one of cellText or cellColours as a parameter to the matplotlib table function is mandatory. These parameters must be 2D lists, in which the outer lists define the rows and the inner list define the column values of each row.
The table can optionally have row and column headers. These optional parameters are configured using rowLabels, rowColours, rowLoc, and colLabels, colColours, colLoc respectively. **kwargs are optional parameters as well.
Parameters:
- cellText: The texts to place into the table cells.
- cellColours: The background colors of the cells.
- cellLoc: The alignment of the text within the cells. (default: ‘right’)
- colWidths: The column widths in units of the axes.
- rowLabels: The text of the row header cells.
- rowColours: The colors of the row header cells.
- rowLoc: The text alignment of the row header cells. (default: ‘left’)
- colLabels: The text of the column header cells.
- colColours: The colors of the column header cells.
- colLoc: The text alignment of the column header cells.(default: ‘left’)
- Loc: This parameter is the position of the cell with respect to ax.
- bbox: This parameter is the bounding box to draw the table into.
- edges: This parameter is the cell edges to be drawn with a line.
- **kwargs: Used to control table properties.
Return type :
The matplotlib.pylot.table() method returns the table created by passing the required condition as parameters.
Implementation of Matplotlib table
import matplotlib.pyplot as plt val1 = ["{:X}".format(i) for i in range(10)] val2 = ["{:02X}".format(10 * i) for i in range(10)] val3 = [["" for c in range(10)] for r in range(10)] fig, ax = plt.subplots() ax.set_axis_off() table = ax.table( cellText = val3, rowLabels = val2, colLabels = val1, rowColours =["palegreen"] * 10, colColours =["palegreen"] * 10, cellLoc ='center', loc ='upper left') ax.set_title('matplotlib.axes.Axes.table() function Example', fontweight ="bold") plt.show()
Output:
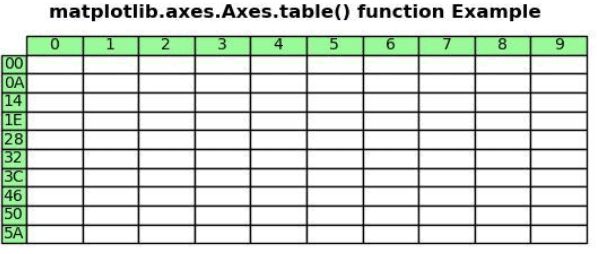
Explanation:
In the above example, desired parameters are passed as arguments to the matplotlib.pyplot.table() to create the table. The parameters passed are as follows:- cellText = val3, rowLabels = val2, colLabels = val1, rowColours =[“palegreen”] * 10, colColours =[“palegreen”] * 10, cellLoc =’center’, loc =’upper left’. Val1, val2 and val3 runs for loops each to generate values for column lables, row lables and cell text, respectively. Setting rowColours and colColours to ‘palegreen’ changes the color of row and column header cells to palegreen. Setting cellLoc =’center’ sets the alignment of header row and column values to center. And finally, loc =’upper left’ sets the alignment of the Title to upper left.
Style Row and Column Headers
rcolors = plt.cm.BuPu(np.full(len(row_headers), 0.1)) ccolors = plt.cm.BuPu(np.full(len(column_headers), 0.1)) the_table = plt.table(cellText=cell_text, rowLabels=row_headers, rowColours=rcolors, rowLoc='right', colColours=ccolors, colLabels=column_headers, loc='center')
Output:
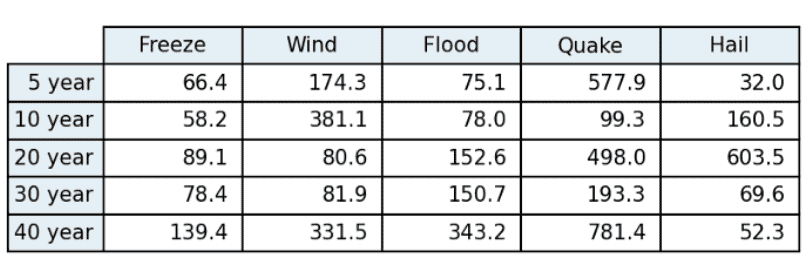
Explanation:
The above code snippet is used to style row and column headers.lists of colors, one for every row header cell and another for every column header cell. Row header horizontal alignment is set to right
. We’ll just use the plt.cm.BuPm
color map to fill two lists of colors, one for every row header cell and another for every column header cell. There are better choices than a linear color map for decorative colors though.
Styling the Matplotlib Table in Python
fig_background_color = 'skyblue' fig_border = 'steelblue' plt.figure(linewidth=2, edgecolor=fig_border, facecolor=fig_background_color ) plt.savefig('pyplot-table-figure-style.png', bbox_inches='tight', edgecolor=fig.get_edgecolor(), facecolor=fig.get_facecolor(), dpi=150 )
Output:
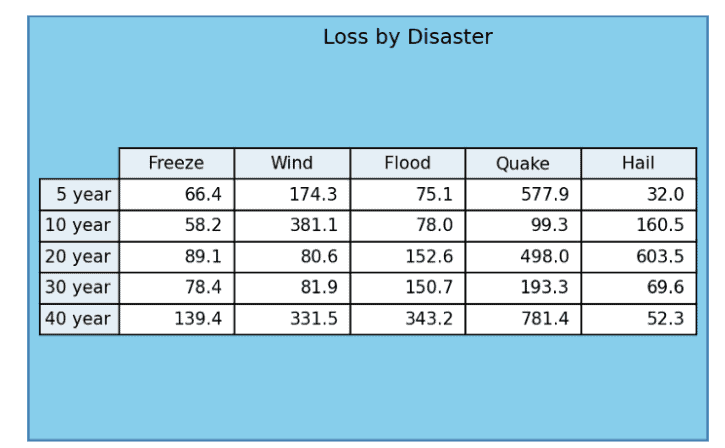
Explanation:
We can explicitly declare the figure to get easy control of its border and background color. In the above code snippet, the background color is set to sky blue. And the figure border is set to steel blue.
Conclusion
This article brings to you very simple and brief concepts of Matplotlib tables in python. It includes ways of inserting tables in your python program in a very neat manner. Methods to style the table and row nd column headers are so explicitly discussed here. Refer to this article for any queries related to tables.
However, if you have any doubts or questions do let me know in the comment section below. I will try to help you as soon as possible.
Happy Pythoning!
The post Matplotlib Table in Python With Examples appeared first on Python Pool.
from Planet Python
via read more
No comments:
Post a Comment