Basic Object-Oriented Programming (OOP) Concepts in Python
In this article you’ll learn the fundamentals of object-oriented programming (OOP) in Python and how to work with classes, objects, and constructors.
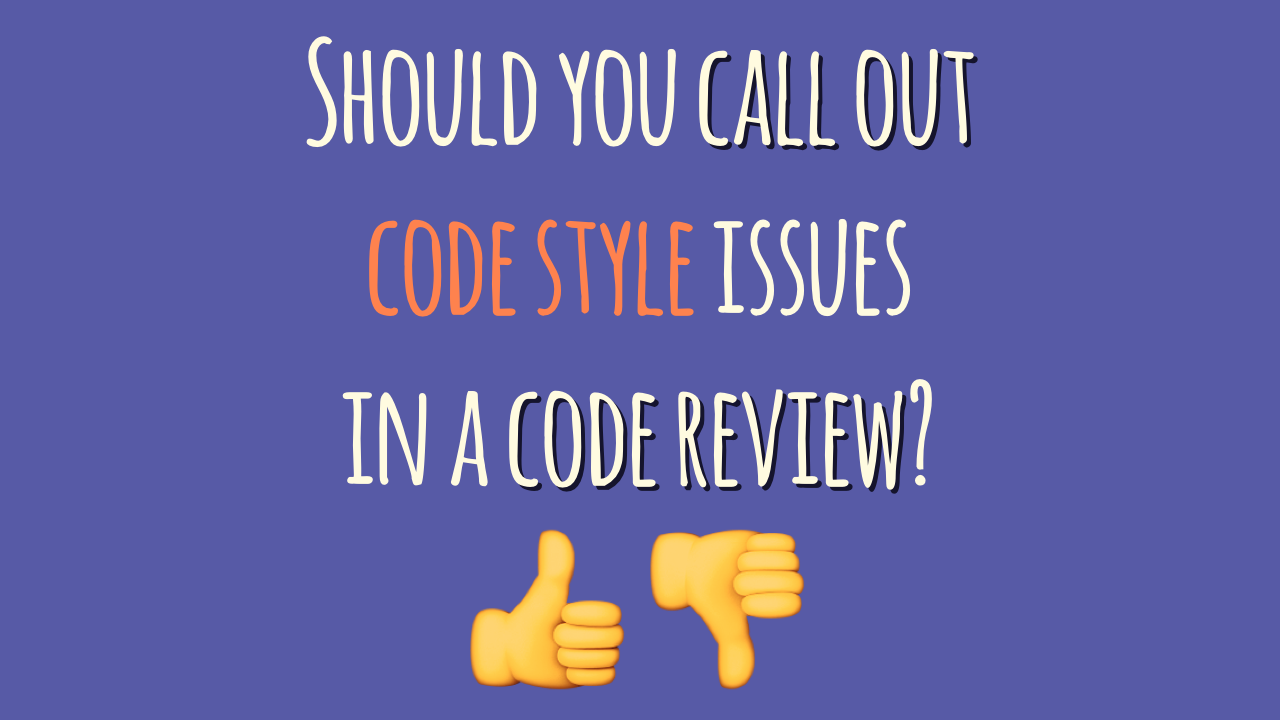
Object-oriented programming (OOP) is a programming paradigm that models real world entities as software objects, which have some data and can perform certain functions.
OOP is best explained with the help of a simple use case:
Suppose you want to develop a car race game using OOP techniques. How would you go about modeling this in your code?
First, you need identify the entities in the game. In simple words, an entity is anything that has some data and can perform certain functions.
In a car race game, for example, a car is an entity: A car has a color, model, price, engine capacity etc. Similarly, a car can perform several functions such as starting, stopping, shift gears, and so on.
Once you have identified the entities in the system you can start implementing them as classes and objects in Python code.
Now, please be aware that OOP is a programming paradigm and not a Python concept. Most of the modern programming languages such as Java, C#, C++ follow OOP principles. So the good news is that learning object-oriented programming fundamentals will be valuable to you in a variety of circumstances—whether you’re working in Python or not.
In this article you’ll pick up the following three basic concepts of OOP in Python:
- Classes
- Object Instances
- Methods
- Inheritance
- Classes
- Objects; and
- Constructors
Let’s start our discussion with the most fundamental of OOP concepts: Classes.
Classes in Python
A class serves as blueprint for the entity that you want to model in your program. Think of a class as analogous to the floor plan of a house—it contains information about the size and locations of the rooms, lawn, parking space, and so on.
But the floor plan isn’t the house, it’s just a plan, a map that encodes the general structure of the house. You’re free to build several houses based on the same single floor plan, for example.
Coming back to our car race game, we said that in this example a car is an entity. However cars can be of multiple sizes, shapes, models, and so forth.
Therefore, we need a blueprint that encodes generic information about a car—a class is the blueprint containing this generic information about a car data structure.
I know, this may sound a little confusing. So let’s take a loot at some real Python code next. We’ll write a simple Car
class in Python 3:
class Car: def __init__(self, name, color): self.name = name self.color = color
Take a careful look at the code above. Let’s see what’s happening here line by line. In the first line of this class definition I tell Python that I’m defining a new class (“entity blueprint”) with the class
keyword, a unique name for the new class, followed by a colon:
class Car: # ...
Right after that comes the class’s constructor. A class constructor in Python is simply a special function called __init__
thats declared in the scope of the surrounding class definition:
class Car: def __init__(self, name, color): # ...
As you can see, the constructor function (__init__
) also accepts three parameters: self
, name
, and color
. Once again the first parameter called self
is special, and the remaining ones (name
and color
) are unique for this example.
Let me explain how this works. When you define a constructor function
class Car: def __init__(self, name, color): self.name = name self.color = color def get_description(self): return "A " + self.color + " " + self.name
class Car: # Attributes name = "" color = "" # Methods def set_name(self, n): self.name = n def set_color(self, c): self.color = c def get_car_info(self): return "This is a " + self.color + " " + self.name
Take a careful look at the code above. Let’s see what’s happening here line by line. In the first line a class Car
is defined. The name of the class follows the class
keyword, followed by a colon:
class Car: # ...
We know that a class has some data and performs functions. In OOP, class data is stored in attributes, and class functions are referred to as methods. After defining the class name, we have defined two attributes name
and `color. The attributes have been initially left empty since a class itself is nothing.
Similarly, four methods named setName, setModel, setColor and getCarInfo have also been defined inside the class. The first three methods set the values of car_name, car_model and car_color variables respectively. The getCarInfo method prints basic information about the car.
If you closely look at the code, we have used “self” keyword both as parameter to the functions as well as inside the function definition. The “self” keyword is used to access the attributes and methods of a class inside the class. Though, the “self” keyword has been used as first parameter inside the function, you don’t have to pass value for this parameter when you call the function. We will see that in the next section.s
At this point of time we have created our Car class. However a class itself is nothing. Class implementation is provided via instances. An object is also called an instance; the two terms are used interchangeably
Python Objects (Instances)
As mentioned earlier, a class only provides a blue print. Class instances or objects actually implement the class. In other words, to use functions and data defined inside a class, you have to create instances of the class. A class can have multiple instances. All the instances have their own copy of data and methods. Let’s create two instances of Car class.
car1 = Car() car1.setName("Honda") car1.setColor("Black") car1.setModel(1999) car1.getCarInfo() car2 = Car() car2.setName("Toyota") car2.setColor("Blue") car2.setModel(2005) car2.getCarInfo()
Executing the above script; you should see the following output:
This is Black Honda ,it is 1999 model This is Blue Toyota ,it is 2005 model
In the script above we create two instances of Car class: car1 and car2. To create an object of a class, (also known as instantiating a class) you simply have to write class name followed by a pair of parenthesis.
By default, we can access class attributes and methods using a function instance. To call a method using instance, you have to write the instance name followed by a dot and the name of the method. Both the instances call the setName, setColor and setModel functions to set different values for the car_name, car_color and car_model attributes. The car_name of car1 instances is set to “Honda” whereas as car_name for the car2 instance is set to “Toyota”. Similarly the remaining attributes also have different values for the two instances. Notice, here we did not pass the value for the “self” parameter of the function, the first parameter is implicitly set as the instance that calls the method.
Now, car1 and car2 instances have their own copies of the attributes as well as the methods of the Car class. To see the value of the attributes of both the instances, the getCarInfo method is called via both the car1 and car2 instances. The getCarInfo method prints the values of the car_name, car_model and car_color attributes. Remember, we set different values for the attributes of both the instances. The output displays different car info, depending upon the attribute values.
Constructors
Constructor is a method that is automatically executed when an instance is created. Typically constructors are used do initialize class attributes. For instance, in the previous section we called the setName, setColor and setColor methods, to set the values of the attributes. We can set these values without calling these methods using constructors. Let’s take a look at a simple example of how constructors are implemented.
class Car: # attributes car_name = "" car_model = 0 car_color = "" # constructor def __init__(self, n, m, c): self.car_name = n self.car_model = m self.car_color = c #methods def getCarInfo(self): print("This is", self.car_color,self.car_name ,",it is", self.car_model, "model")
The __init__
method of a class serves as its constructor. This is unlike other modern programming languages where constructor method has the name same as the name of the class. In python __init__
method is automatically called when a class is instantiate. (Creating a class object refers to instantiating a class). In the above script, the constructor takes three parameters in addition to “self”. This constructor sets the values of the instance attributes to the values passed in the parameters. When you create the object of the Car class, you will have to pass the values for these parameters.
Now let’s us see how to pass the arguments to the Car class constructor. Execute the following script:
car1 = Car("Audi",2006,"Black") car1.getCarInfo() car2 = Car("Ferari",2017,"Red") car2.getCarInfo()
The arguments for the constructor are passed within the parenthesis while instantiating a class. In the script above, we create two Car objects: car1 and car2. For the car1 object we passed “Audi”, 2006 and “Black” as parameters for the Car constructor. When the car1 object is created, the Car constructor executes and sets the values of car_name, car_model and car_color attributes to the three values passed in the constructor. To see if the attribute values have been set, we call the getCarInfo method which prints the attribute values. Similarly we create car2 object and pass values for its constructor too and then call getCarInfo method of the car2 object. In the output, you will see the values for the attributes of both the objects. The output will look like this:
This is Black Audi ,it is 2006 model This is Red Ferari ,it is 2017 model
OOP in Python – Conclusion
This article provides a brief introduction to object oriented. We study what are the classes and objects and how they are created. We also saw how constructors are used to initialize the class attributes. However, this is just tip of the iceberg. OOP is a very vast subject and cannot be covered in a single article. In the upcoming articles, we shall explore some other important OOP concepts such as Inheritance, Encapsulation, and Polymorphism etc.
- Python classes allow you to create new types of variables (instances), with built-in functions, using a simple syntax. Another way to think of classes is as “object instance factories.”
[ Improve Your Python with Dan's 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
from Planet Python
via read more
No comments:
Post a Comment