The post How to Convert a Float Array to an Integer Array in Python with NumPy appeared first on Erik Marsja.
In this short NumPy tutorial, we are going to learn how to convert a float array to an integer array in Python. Specifically, here we are going to learn by example how to carry out this rather simple conversion task. First, we are going to change the data type from float to integer in a 1-dimensional array. Second, we are going to convert float to integer in a 2-dimensional array.
Now, sometimes we may want to round the numbers before we change the data type. Thus, we are going through a couple of examples, as well, in which we 1) round the numbers with the round()
method, 2) round the numbers to the nearest largest in with the ceil()
method, 3) round the float numbers to the nearest smallest numbers with floor()
method. Note, all code can be found in a Jupyter Notebook.
Creating a Float Array
First, however, we are going to create an example NumPy 1-dimensional array:
import numpy as np # Creating a 1-d array with float numbers oned = np.array([0.1, 0.3, 0.4, 0.6, -1.1, 0.3])
As you can see, in the code chunk above, we started by importing NumPy as np. Second, we created a 1-dimensional array with the array()
method. Here’s the output of the array containing float numbers:
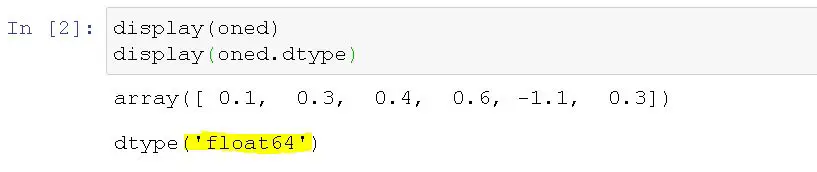
Now, we are also going to be converting a 2-dimensional array so let’s create this one as well:
# Creating a 2-d float array twod = np.array([[ 0.3, 1.2, 2.4, 3.1, 4.3], [ 5.9, 6.8, 7.6, 8.5, 9.2], [10.11, 11.1, 12.23, 13.2, 14.2], [15.2, 16.4, 17.1, 18.1, 19.1]])
Note, if you have imported your data with Pandas you can also convert the dataframe to a NumPy array. In the next section, we will be converting the 1-dimensional array to integer data type using the astype() method.
How to Convert a Float Array to an Integer Array in Python:
Here’s how to convert a float array to an integer array in Python:
# convert array to integer python oned_int = oned.astype(int)
Now, if we want to change the data type (i.e. from float to int) in the 2-dimensional array we will do as follows:
# python convert array to int twod_int = twod.astype(int)
Now, in the output, from both conversion examples above, we can see that the float numbers were rounded down. In some cases, we may want the float numbers to be rounded according to common practice. Therefore, in the next section, we are going to use around()
method before converting.
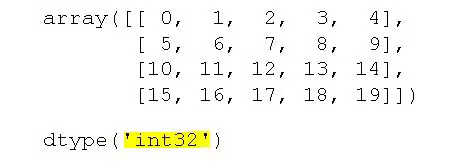
Now, if we want to we can now convert the NumPy array to Pandas dataframe, as well as carrying out descriptive statistics.
Round the Float Numbers Before we Convert them to Integer
Here’s how to use the around()
method before converting the float array to an integer array:
oned = np.array([0.1, 0.3, 0.4, 0.6, -1.1, 0.3]) oned = np.around(oned) # numpy convert to int oned_int = oned.astype(int)
Now, we can see in the output that the float numbers are rounded up when they should be and, then, we converted them to integers. Here’s the output of the converted array:
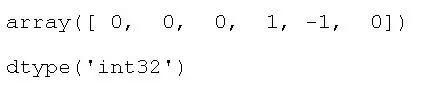
Round to the Nearest Largest Integer Before Converting to Int
Here’s how we can use the ceil() method before converting the array to integer:
oned = np.array([0.1, 0.3, 0.4, 0.6, -1.1, 0.3]) oned = np.ceil(oned) # numpy float to int oned_int = oned.astype(int)
Now, we can see the different in the output containing the converted float numbers:
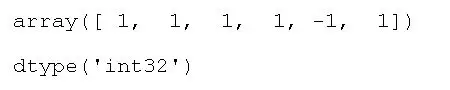
Round to the Nearest Smallest Integer Before Converting to Int
Here’s how to round the numbers to the smallest integer and changing the data type from float to integer:
oned = np.array([0.1, 0.3, 0.4, 0.6, -1.1, 0.3]) oned = np.floor(oned) # numpy float to int oned_int = oned.astype(int)
In the image below, we see the results of using the floor() method before converting the array. It is, of course, possible to carry out the rounding task before converting a 2-dimensional float array to integer, as well.
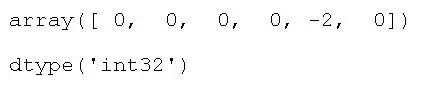
Here’s the link to the Jupyter Notebook containing all the code examples found in this post.
Conclusion
In this NumPy tutorial, we have learned a simple conversion task. That is, we have converted a float array to an integer array. To change the data type of the array we used the astype()
method. Hope you learned something. Please share the post across your social media accounts if you did! Support the blog by becoming a patron. Finally, if you have any suggestions, comments, or anything you want me to cover in the blog: leave a comment below.
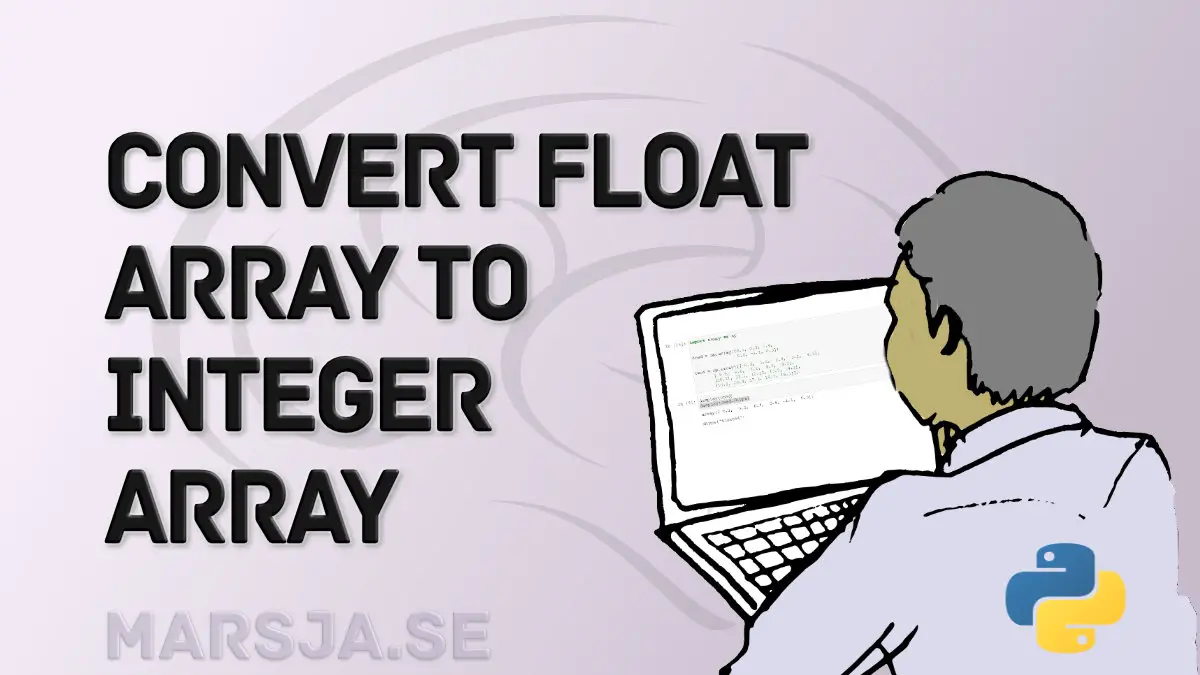
The post How to Convert a Float Array to an Integer Array in Python with NumPy appeared first on Erik Marsja.
from Planet Python
via read more
No comments:
Post a Comment