The post How to get Absolute Value in Python with abs() and Pandas appeared first on Erik Marsja.
In this Python tutorial, we will learn how to get the absolute value in Python. First, we will use the function abs() to do this. In this section, we will go through a couple of examples of how to get the absolute value. Second, we will import data with Pandas and use the abs method to get the absolute values in a Pandas dataframe.
Python Absolute Value Tutorial
Now, before we go on with the examples on how to get the absolute value of a number using Python, we will go quickly into what absolute value is:
What is an absolute value?
Pretty simple; it means how far a value is from zero.
How do I get the absolute value in Python?
Python abs() Function
The Python abs() function is one of the math functions in Python. This function will return the positive absolute value of a specific number or an expression. In the next sections, we will see plenty of examples of how to get the absolute value in Python. First, however, we are going to have a look at the syntax of the abs() function.
Python abs syntax
The syntax of the abs() function in the Python programming language is as shown below:
abs(x)
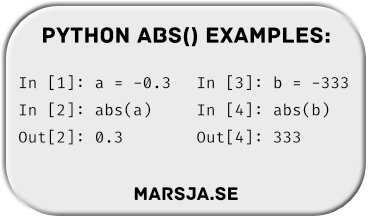
Now, x can be any number that we want to find the absolute value for. For instance, if x is positive or negative zero, Pythons abs() function will return positive zero.
If we, however, put in something that is not a number we will get a TypeError (“bad operand type for abs(): ‘str’”.
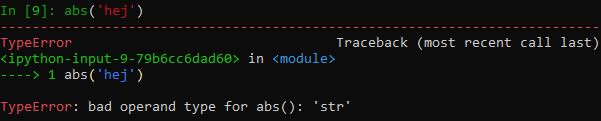
How to get Absolute Value in Python with abs() Example 1
The abs function will enable us to find the absolute value of a numeric value. In this how-to get absolute value in Python example, we are going to find the absolute values of different data and display the output.
abs(-33)
Python abs() Example 2
Now, if we have a list of numbers, we cannot use the abs() function as we did in the first example. Note, if we do we get a TypeError, again. Thus, in this example, we are going to use Python list comprehension and the abs() function.
numbers = [-1, -2.1, -3, -444]
[abs(number) for number in numbers]
Note, it is also possible to import the math module and use the fabs() method to get the absolute value of a number in Python. However, when using fabs(), we will get the absolute value as a float:
import math
math.fabs(-33)
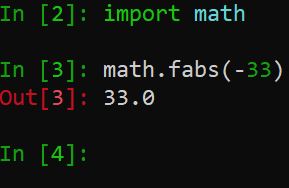
Python get Absolute Values in Python using Pandas
Now, if we want to get absolute values from a dataset we can use Pandas to create a dataframe from a datafile (e.g., CSV). In this Python absolute value example, we are going to find the absolute values for all the records present in a dataframe. First, we will use Python to get absolute values in one column using Pandas abs method. Second, we will do the same but this for two columns in the dataset. Finally, we will get the absolute values for all columns in the Pandas dataframe.
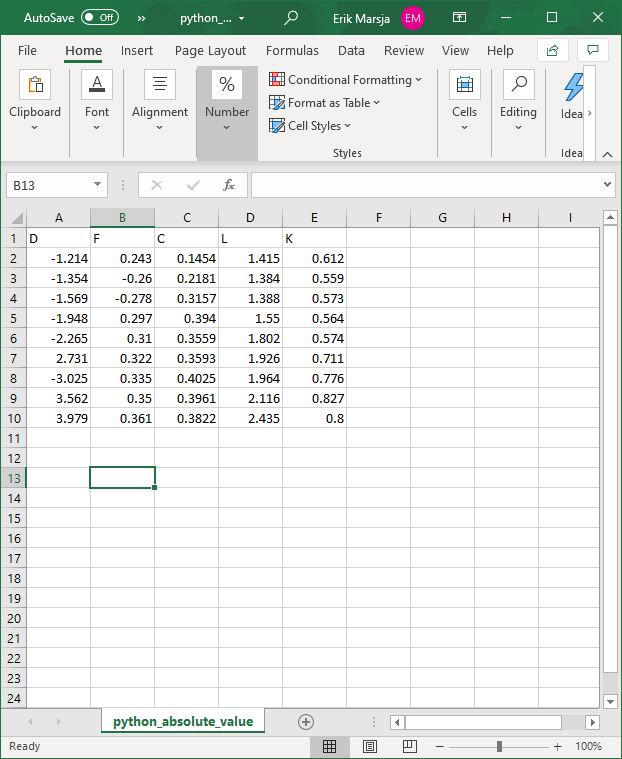
Now, for this Python absolute value example, we are going to use the CSV data in the image above. If needed, see the post about Pandas read csv method to understand the steps in importing data from a CSV file. Here’s how to do it, with the example file (python_absolute_value.csv):
import pandas as pd
df = pd.read_csv('python_absolute_value.csv')
Now, when we have the data loaded we are ready to get the absolute values using Python Pandas.
Python Pandas Absolute Values Example 1
In the first Python absolute values example using Pandas, we are going to select one column (“D”):
df['D'].abs()
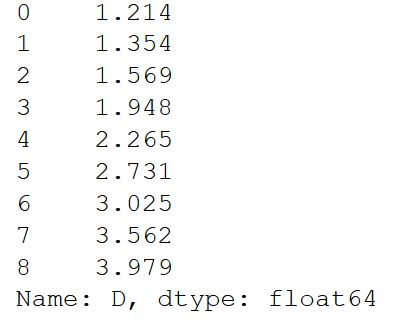
Python Pandas Absolute Values Example 2
Now, in the second absolute values example, we are going to select two columns (“D” and “F”):
df[['D', 'F']].abs()
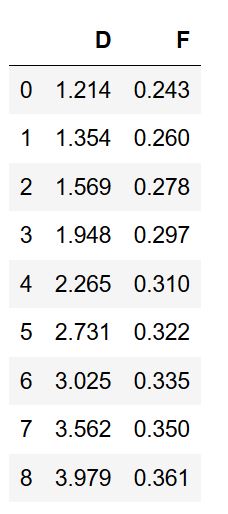
Now, if needed there’s more information about slicing and indexing Pandas dataframes in that post.
Python Pandas Absolute Values Example 3
Finally, we are going to get the absolute values from all columns in the Pandas dataframe:
df.abs()
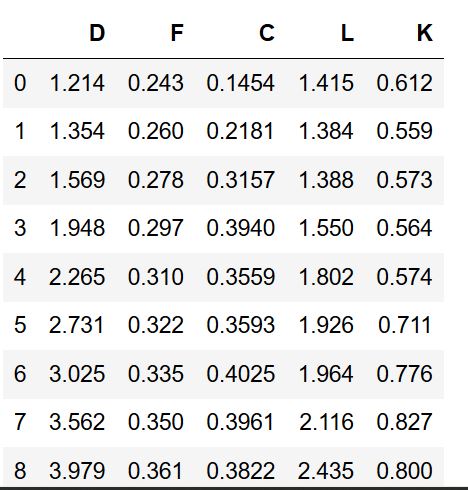
Conclusion: Python Absolute Value
Now, in this post, we learned how to get the absolute value in Python. It was pretty simple, we just used the abs() function. Second, we learned how to do the same task but with data stored on our computers (e.g., from a CSV file).
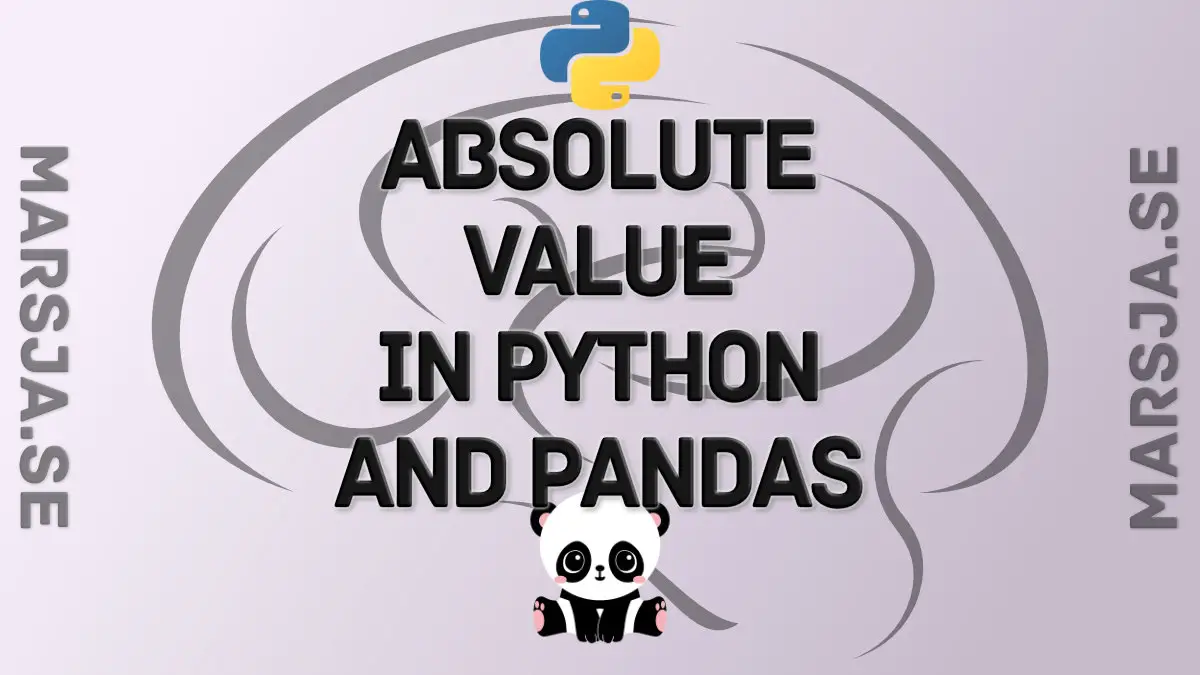
The post How to get Absolute Value in Python with abs() and Pandas appeared first on Erik Marsja.
from Planet Python
via read more
No comments:
Post a Comment