The post How to Get the Column Names from a Pandas Dataframe – Print and List appeared first on Erik Marsja.
In this short post, we will learn 6 methods to get the column names from Pandas dataframe. One of the nice things about Pandas dataframes is that each column will have a name (i.e., the variables in the dataset). Now, we can use these names to access specific columns by name without having to know which column number it is.
To access the names of a Pandas dataframe, we can the method columns(). For example, if our dataframe is called df we just type print(df.columns) to get all the columns of the pandas dataframe.
After this, we can work with the columns to access certain columns, rename a column, and so on.
Importing Data from a CSV File
First, before learning the 6 methods to obtain the column names in Pandas, we need some example data. In this post, we will use Pandas read_csv to import data from a CSV file (from this URL). Now, the first step is, as usual, when working with Pandas to import Pandas as pd.
import pandas as pd
df = pd.read_csv('https://vincentarelbundock.github.io/Rdatasets/csv/carData/UN98.csv',
index_col=0)
df.head()
It is, of course, also possible to read xlsx files using Pandas read_excel method.
Six Methods to Get the Column Names from Pandas Dataframe:
Now, we are ready to learn how we can get all the names using different methods.
1. Get the Names Using the columns method
Now, one of the simplest methods to get all the columns from a Pandas dataframe is, of course, using the columns method and printing it. In the code chunk below, we are doing exactly this.
print(df.columns)
2. Access Column Names Using the keys() Method
Second, we can get the exact same result by using the keys() method. That is, we will get the column names by the following code as well.
print(df.keys())
3. Get Column Names by Iterating of the Columns
In the third method, we will simply iterate over the columns to get the column names. As you may notice, we are again using the columns method.
for col_name in df.columns:
print(col_name)
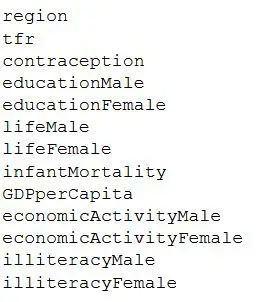
4. Get the Column Names as a List
In the fourth method, on the other hand, we are going to use the list() method to print the column names as a list.
print(list(df.columns))

5. Another Method to Print Column Names as a List
Now, we can use the values method, as well, to get the columns from Pandas dataframe. If we also use the tolist() method, we will get a list, as well.
print(df.columns.values.tolist())
6. How to Get the Column Names with Pandas Sorted
Now, in the final, and sixth, method to print the names, we will use sorted() to get the columns from a Pandas dataframe in alphabetic order:
sorted(df)
How to Get Values by Column Name:
Now, that we know the column names of our dataframe we can access one column (or many). Here’s how we get the values from one column:
print(df['tfr'].values)
If we, on the other hand, want to access more than one column we add a list: df[['tfr', 'region']]
How to Rename a Column
In the final example, on what we can do when we know the column names of a Pandas dataframe is to rename a column.
df.rename(columns={'tfr': 'TFR'})
Note, if we want to save the changed name to our dataframe we can add the inplace=True, to the code above.
Conclusion: Getting all the Column Names with Pandas
Now, in this post, we have learned how to get the column names from a Pandas dataframe. Specifically, we learned why and when this can be useful, 6 different methods to access the column names, and very briefly what we can do when we know the column names. Finally, here’s the Jupyter Notebook with all the example code.
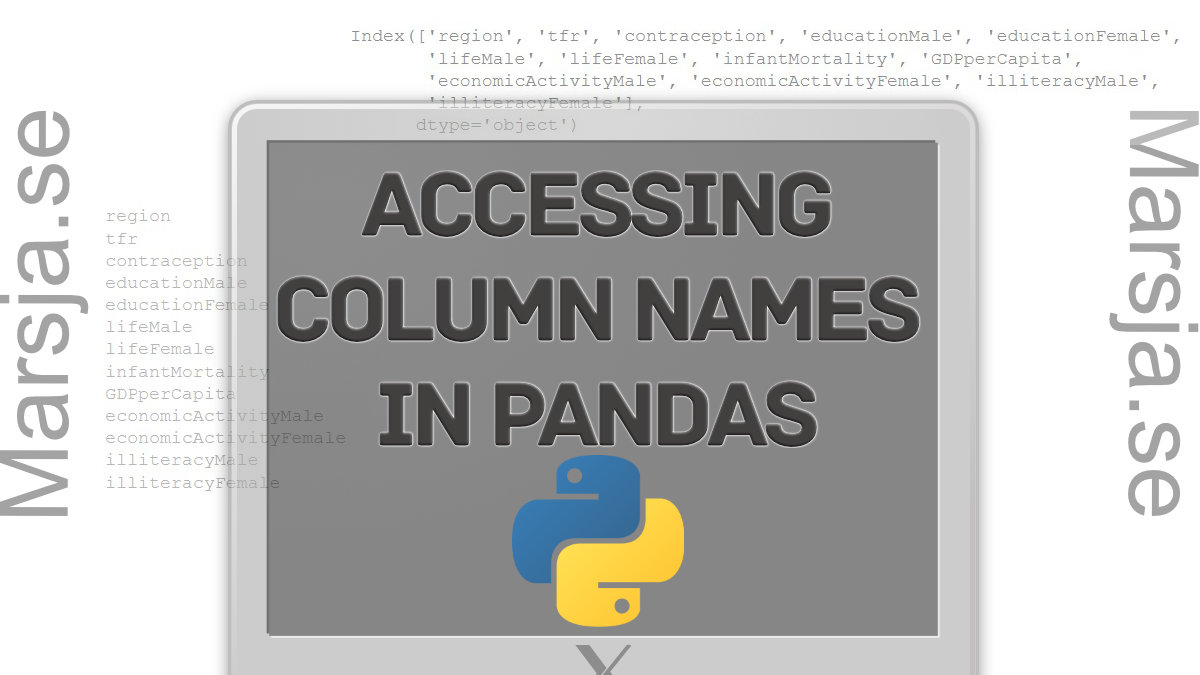
The post How to Get the Column Names from a Pandas Dataframe – Print and List appeared first on Erik Marsja.
from Planet Python
via read more
No comments:
Post a Comment