The post Rename Files in Python: A Guide with Examples using os.rename() appeared first on Erik Marsja.
In this post, we are going to work with Python 3 to rename files. Specifically, we will use the Python module os to rename a file and rename multiple files.
First, we will rename a single file in 4 easy steps. After that, we will learn how to rename multiple files using Python 3. To be able to change the name of multiple files using Python can come in handy. For example, if we have a bunch of data files (e.g., .csv files) with long, or strange names, we may want to rename them to make working with them easier later in our projects (e.g., when loading the CSV files into a Pandas dataframe).
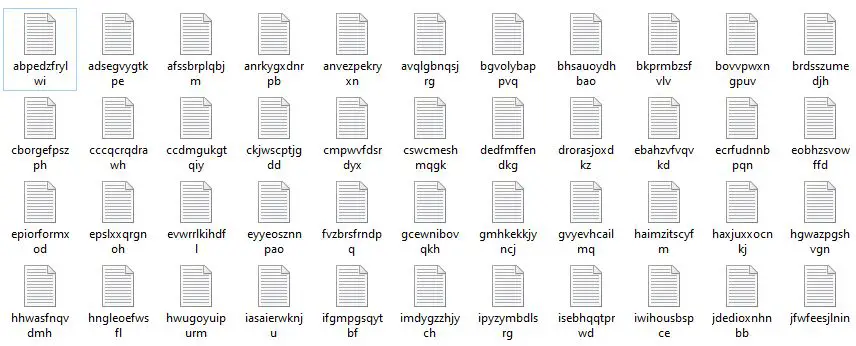
How to Rename a File in Python
For instance, if we have a file called python-rename-files.txt we need to know where we have stored it. There are, of course, different ways to do this. Either, we can place the Python script in the same directory and just run it to rename the file. Here’s a simple example on how to rename a file in Python:
import os
os.rename('python-rename-files.txt', 'renamed-file.txt')
4 Simple Steps to Rename a File in Python
In the first section, we are going to learn how to rename a single file in Python step-by-step. Now, the general procedure is similar when we are using Linux or Windows. However, how we go about in the first step to rename a file in Python may differ depending on which OS we use. In the renaming a file in Python examples below, we will learn how to carry on and changing names both in Linux and Windows.
1. Getting the File Path of the File we Want to Rename With Python
First, to get Python to rename a file Python needs to know where the file is located. That is, step 1 is finding the location of the file we want to change the name on.
That is, if we store our Python scripts (or Jupyter notebooks) in certain directories, we need to tell Python the complete path to the file we want to rename.
Finding the File Path in Windows
If we use Windows, we can open up the File Explorer. First, go to the folder where the file is located (e.g., “Files_To_Rename”) and click on the file path (see image below) It should look something like “C:\Users\erima96\Documents\Files_To_Rename”.
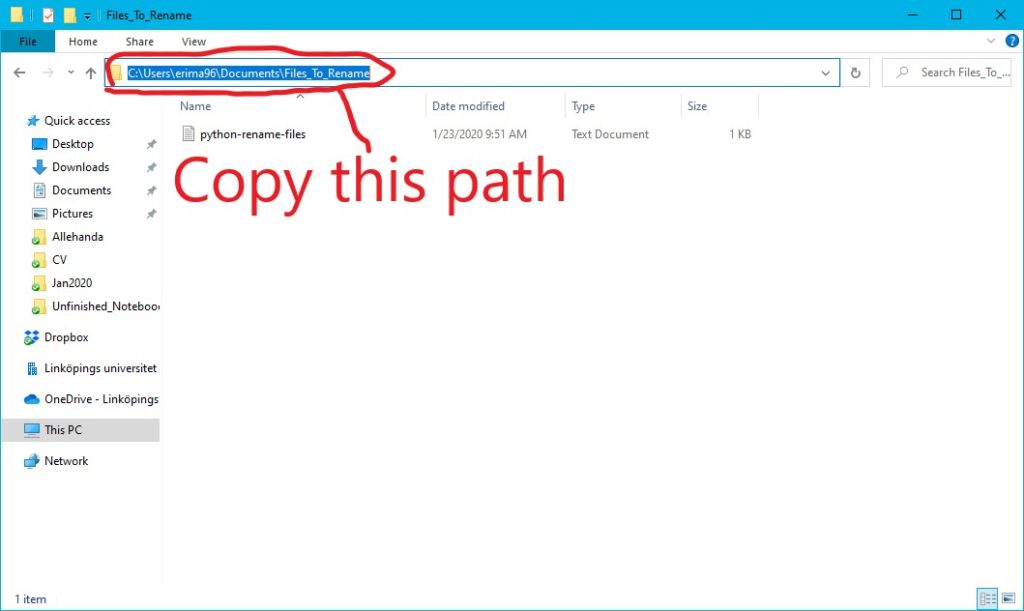
Finding the File Path in Linux
Now, if we are to rename a file, using Python, in Linux we need to know the path. There are, of course, many methods to find the file path in Linux. One method is to open up a Terminal Window and use the readlink and xclip command-line applications:
readlink -f FILE_AND PATH | xclip -i
For instance, if the file we want to rename with Python is located in the folder “/home/jhf/Documents/Files_To_Rename/python-rename-files.txt” this is how we would do it:
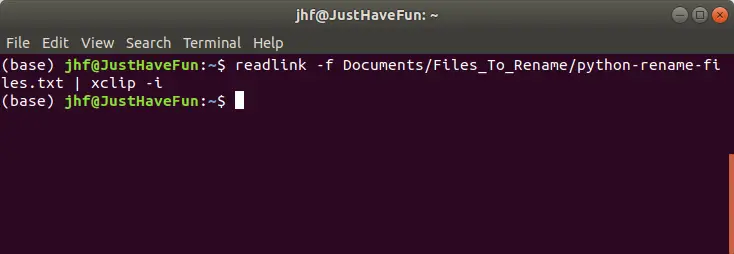
2. Copy the Path of the File to Rename
Second, we copy the path of the file we want to rename. Note, this is as simple as just right-clicking on the path we already have marked:
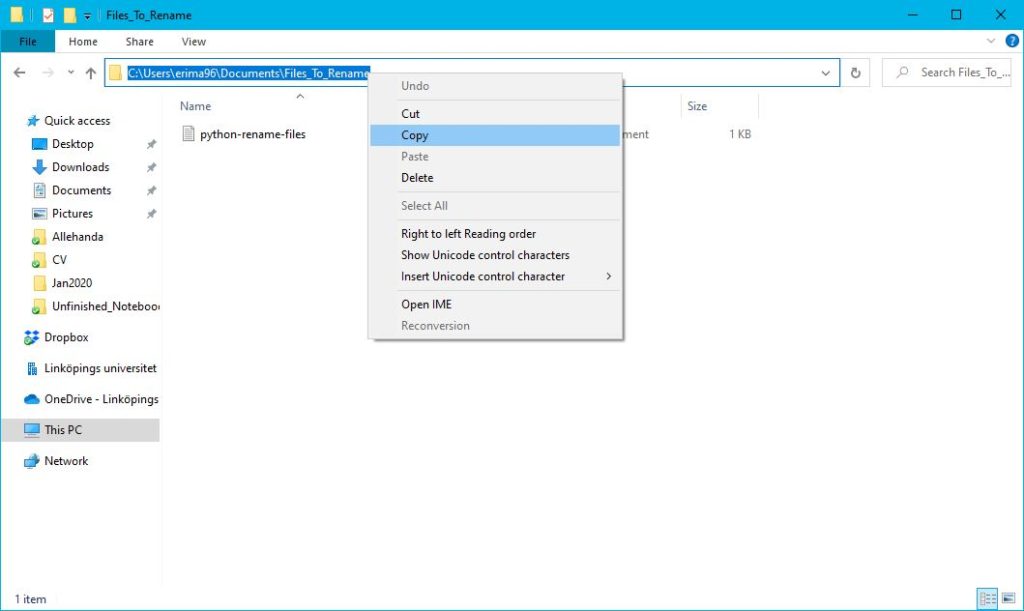
3. Importing the os Module
Third, we need to import a Python module called os. Luckily, this is very easy and we just type: import os
. In the next step, we will actually rename a file using Python!
4. Renaming the File
Finally, we are ready to change the name of the file using the os module from Python. As the file path can be long we will create a string variable, fist, and then rename the file using os.rename. Here’s how to rename a file using Python:os.rename(PATH_TO_THE_FILE, PATH_TO_THE_NEW_FILE)
Changing a Name of a File in Windows
Here’s a full code example, for Windows users, on how to change the name of a file using Python:
import os
file_path = 'C:\\Users\\erima96\\Documents\\Files_To_Rename\\'
os.rename(file_path + 'renamed-file.txt',
file_path + 'python-rename-files.txt')
Note, how we added an extra ”\” to each subfolder (and at the end). In the os.rename method we also added the file_path to both the file we want to rename and the new file name (the string variable with the path to the file) and added the file name using ‘+’. If we don’t do this the file will also be moved to the folder of the Python script. If we run the above code, we can see that we have successfully renamed the file using Python:
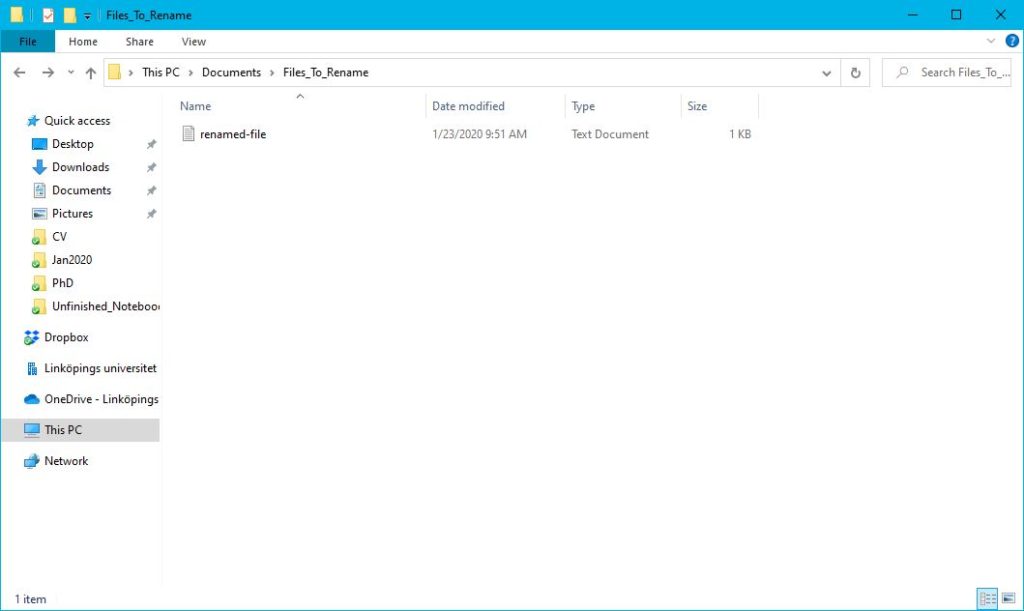
Renaming a File in Linux
Here’s how to rename the file in Linux. Note, the file path will be a bit different (as we saw earlier when we found the file path):
import os
file_path = '/home/jhf/Documents/Files_To_Rename/python-rename-files.txt'
os.rename(file_path + 'renamed-file.txt',
file_path + 'python-rename-files.txt')
Now that we have learned how to rename a file in Python we are going to continue with an example in which we change the name of multiple files. As previously mentioned, renaming multiple files can come in handy if we have many files that need new names.
How to Rename Multiple Files in Python
In this section, we are going to learn how to rename multiple files in Python. There are, of course, several ways to do this. One method could be to create a Python list with all the file names that we want to change. However, if we have many files this is not an optimal way to change the name of multiple files. Thus, we are going to use another method from the os module; listdir. Furthermore, we will also use the filter method from the module called fnmatch.
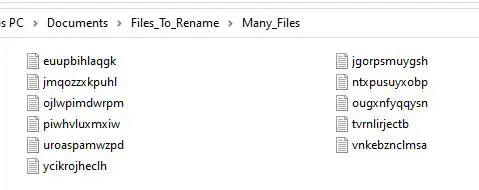
The first step, before renaming the files, is to create a list of all text files in a folder (e.g., in the subfolder “Many_Files”). Note, that we have added the subfolder to the file_path string.
import os, fnmatch
file_path = 'C:\\Users\\erima96\\Documents\\Files_To_Rename\\Many_Files\\'
files_to_rename = fnmatch.filter(os.listdir(file_path), '*.txt')
print(files_to_rename)

Next, we are going to loop through each file name and rename them. In the example below, we are using the file_path string, from the code chunk above, because this is where the files are located. If we were to have the files we want to rename in a different folder, we’d change file_path to that folder. Furthermore, we are creating a new file name (e.g., new_name = ‘new_file’) and we use the enumerate method to give them a unique name. For example, the file ‘euupbihlaqgk.txt’ will be renamed ‘Datafile1.txt’.
code class="lang-py">new_name = 'Datafile' for i, file_name in enumerate(files_to_rename): new_file_name = new_name + str(i) + '.txt' os.rename(file_path + file_name, file_path + new_file_name)
As can be seen in the rename a file in Python example above, we also used the str() method to change the data type of the integer variable called i to a string.
Remember, if we were using Linux we’d have to change the file_path so that it will find the files we want to rename.
Renaming Multiple Files by Replacing Characters in the File Name
Now, sometimes we have files that we want to replace certain characters from. In this final example, we are going to use the replace method to replace underscores (“_”) from file names. This, of course, means that we need to rename the files.
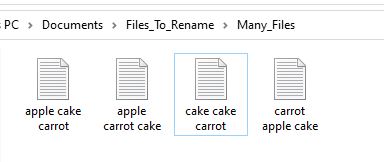
code class="lang-py">import os, fnmatch file_path = 'C:\\Users\\erima96\\Documents\\Files_To_Rename\\Many_Files\\' files_to_rename = fnmatch.filter(os.listdir(file_path), '*.txt') for file_name in files_to_rename: os.rename(file_path + file_name, file_path + file_name.replace(' ', '-'))
Now, in the code above we have successfully replaced the “_” from the filenames using replace and then we renamed the files. Again, remember if we were to rename files in Linux the file_path string need be changed (e.g. ” “).
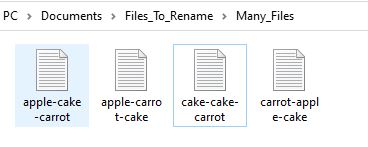
Conclusion: Renaming Files in Python
In this post, we have learned how to rename a single file, as well as multiple files, using Python. This was accomplished using the os and fnmatch modules. Finally, we learned how to replace underscores (and rename) files using Python.
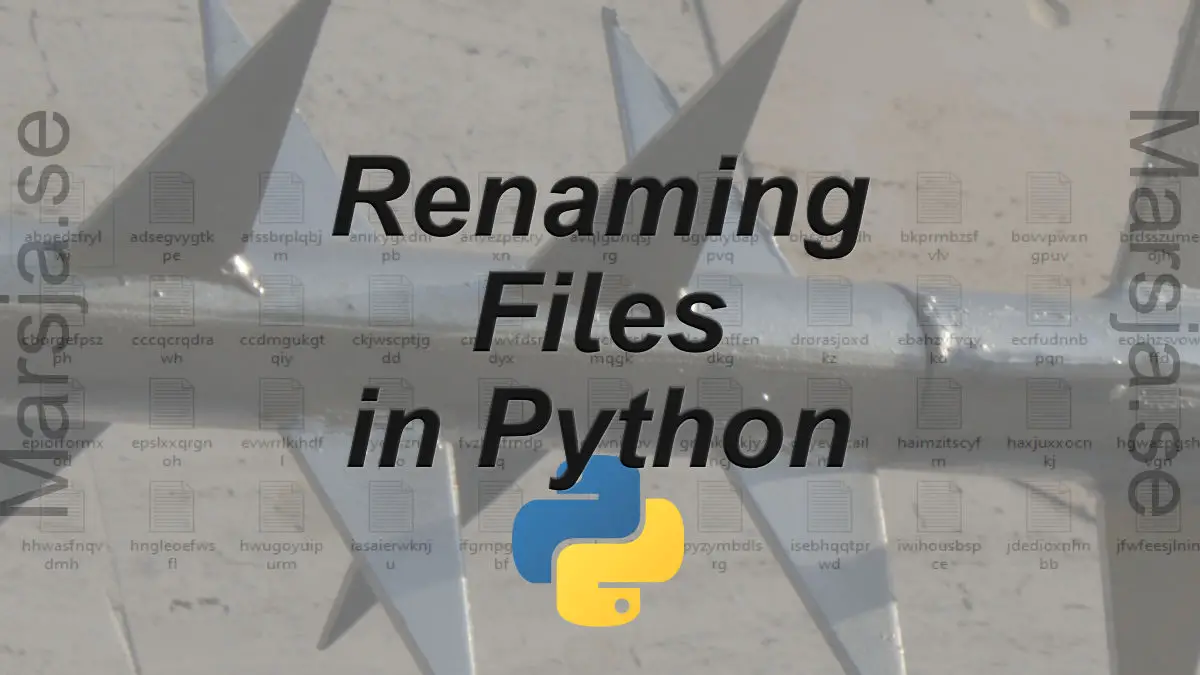
The post Rename Files in Python: A Guide with Examples using os.rename() appeared first on Erik Marsja.
from Planet Python
via read more
No comments:
Post a Comment